Important C Programs – A Perfect Collection of important Basic to Advanced Level C Programs for Beginners, very helpful for learning C Language easily!
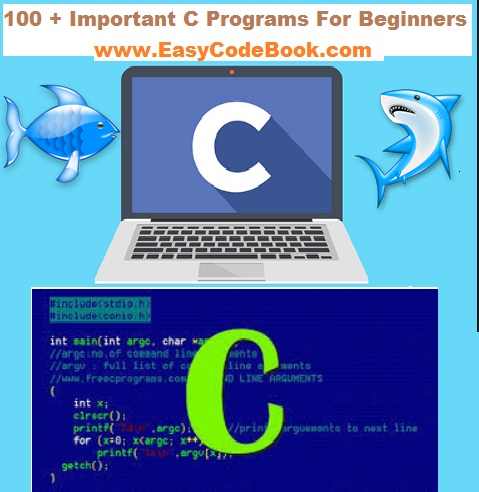
100 + Important C Programs for Beginners
C Language Programs
Here are 100 + C programs to improve your programming skills in C language. The C language programs provided here are very easy to understand. They will cover the following important topics thoroughly. You will enjoy the reading indeed!
More and more C Language programs will be published on this page soon! Keep visiting www.easycodebook.com. Enjoy your stay here! Welcome to the C Programming World!
If you find this programming page helpful, please share it on social media like Facebook, Pinterest, Twitter and Instagram. Thank You!
1. Basic C Programs
Here is a bunch of basic programs to start learning programming skills. These are mostly simple formula calculation programs. In such basic programs, we learn to use expressions, assignment statement and basic input / output statements.
- Print Hello World
- Add two numbers
- Convert Miles to Kilometers KM
- Convert Kilometers Km to Meters
- Swap two Variable’s values using Third variable
- Exchange two varibles values without third variable
- Swap values of two variables using XOR
- Convert Meter to Feet
- Convert Feet to Meters
- Temperature Converter Celcius to Fahrenheit
- Convert Fahrenheit to celcius Temperature
- Person Height from Inches to Centimeters
- Convert mm milimeters to inches and More Programs
2. Conditional C Programs
The conditional Logic is very important for beginner programmers. Here we will learn the use of if statement, if-else statement, if-else-if statement, nested if statement and switch statemnt.
- Check Both Numbers are Equal if else
- Larger between two variables
- Largest of two numbers using Ternary Operator
- Greatest among 3 using if and Logical Operators
- Largest value among 3 using Ternary Operator
- Find / Check Leap Year
- Even Odd Number
- Even Odd Number using Ternary Operator
- Positive Negative or Zero
- Simple if to check larger number or equal
- Four Function Calculator using switch statement and More programs
3. Loop C Program
The C language Provides three types of loops. These looping statements are used to execute a set or block of statements as long as a given condition is satisfied.
There are three types of loops in C Programming:
for Loop
while Loop
do-while Loop
- Print 1 to 10 Numbers
- Display First 10 Natural Numbers
- Factorial of N
- Power – N Raised to Power P
- Find Sum of Digits of N
- Reverse a Number
- Check Palindrome Number
- Fibonacci Number Series
- Check Prime Number or Composite
- Check Armstrong Number
- Binary to Decimal Number Converter
- Print Floyd’s Triangle
- Find GCD of Two Numbers
- Find LCM of Two Numbers
- FIND GCD and LCM
- and More programs
4. Array C Programs
The arrays in C programming are very important to handle a group of data of same type. For example, if we wish to calculate average temperature of the city for the whole month of March, we will use an array of 31 float numbers.
- Maximum / Largest Number of Array
- Minimum / Smallest value of Array
- Display Reverse Array
- Array Input and Output Elements
- Input of Elements in Float Array
- Find Average Temperature of Week
- Search an Item in Array
- Sort Array of N Numbers
- Find Count f Occurrences of each element of array ( frequencies of numbers in array ) and More Programs
5. Matrix ( Two dimensional array ) C Programs
A Matrix is a two dimensional array. In C language programs, we can use a matrix to manipulate data in tables that is in rows and columns. The following are some of the programs solved using matrix – 2D array.
- Matrix Input Output – 2D Array input output
- Sum of two Matrix ( Matrices ) 2 Dimentional arrays
- Subtract two matrices (matrix)
- Matrix Transpose(Swapping Rows and Columns)
- Matrix Multiplication – Multiply Two Matrices
- Sum of Main Diagonal Elements of Square Matrix
- and More programs
6. Star Pattern Programs / Pyramids
- Printing star diamond pattern
- Show star pattern center down triangle
- Star triangle center pattern
- Print star pattern triangle upside down
- Star pattern Right Angle Triangle
- Print Star Pattern Left Inverted Triangle
- Display Star Pattern Triangle using loop
- C Program Print Heart Shape Star Pattern
- + More programs
7. Numbers Pattern Programs / Pyramids
- Square Number Pattern in C Programming
- Print Number Pattern Square Shape 5 to 1
- Printing Number Square Pattern in C Programming
- Display Number Triangle Pattern 1 to 5
- Number Triangle Pattern in C Programming
- Number Pattern Left Triangle in C Programming
- + more
8. Alphabets Patterns C Programs
- Continuous alphabets Pattern in C Programming
- Shapes with Alphabets 2 in C Programming
- Alphabet Triangle Pattern in C Programming
- + More programs
9. String C Programs
- Sort Array of Strings- Sort N Strings alphabetically
- Find String Length – strlen()
- Convert String to Lower Case – strlwr()
- Convert String to UPPER CASE – strupr()
- Toggle case Conversion of String
- String Compare – strcmp()
- String Compare Ignore case – stricmp() case insensitive search
- Reverse String- strrev()
- Concatenation of strings- strcat()
- Search word position in string – strstr()
- Remove Blank Spaces from String C Program
- Count Digits in String C Program
- Count Words in String C Program
- Check Palindrome String C Program
- and More Programs…
10. Recursion and C Function Programs
- Factorial by Recursion
- Power by Recursion
- Fibonacci Number series by Recursion
- Reverse a Number by Recursion
- Sum of Digits by Recursion
- GCD by Recursion and More Programs
11. Searching and Sorting C Programs
The searching problems are very important area of coding in any programming language. In this C language tutorial and the solved exeecises of c programming, the Linear search algorithm and The binary search algorithm is the most important. You will find these programs in the following C Programming section.
Sorting a list of numbers either in ascending order or in descending order is another poular programming technique. Here are five the most popular sorting programs in C Language witheasy logic.
- Quick Sort
- Merge Sort
- Bubble Sort
- Selection Sort
- Insertion Sort and More Programs …
12. File C Programs
The files are important to store data created and manipulated by C programs, permanently on the disk. Especially, storing records in a file is one of the most challenging programming assignments in computer programming.
- Write Records in Binary File
- Read Records from Binary File
- Append / add more records in binary file
- Search a Particular Record from File
- Search a Record by Name
- Find Multiple Records in a file according to given criteria + More Programs in C filing
13. Calendar / Date / Time Program in C Language