Topic: Display Yearly Calendar C Program
First of all this c program will require a four digit year to input. As soon as the use enters a correct year for example, 2020, this c program will display calendar of the year 2020 month wise. This c program to display calendar uses smaller modules called functions to divide the logic of the program. Sso that the beginner programmers can understand the logic of the C calendar Program easily.
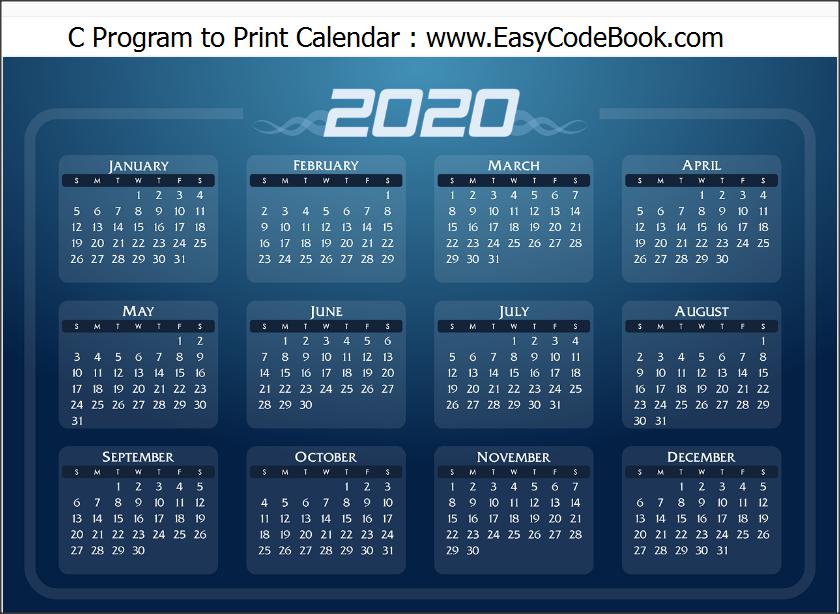
C Program to print calendar of a given year month wise
/* Write a C Language Program To Input a four digit year like 2020 and Print the whole year's print_calendar, month wise */ #include<stdio.h> int month_days[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; char *month_name[]= { " ", "\n\n\nJanuary", "\n\n\nFebruary", "\n\n\nMarch", "\n\n\nApril", "\n\n\nMay", "\n\n\nJune", "\n\n\nJuly", "\n\n\nAugust", "\n\n\nSeptember", "\n\n\nOctober", "\n\n\nNovember", "\n\n\nDecember" }; /* This functions returns daycode of the first day of the year. if daycode is 1, first day is Monday */ int get_first_daycode(int year) { int daycode; int x, y, z; x = (year - 1) / 4; y = (year - 1) / 100; z = (year - 1) / 400; daycode = (year + x - y + z) %7; return daycode; } void set_feb_days(int year) { if(year% 4 == 0 && year%100 != 0 || year%400 == 0) month_days[2] = 29; else month_days[2] = 28; } void print_calendar(int year, int daycode) { int month, day; for ( month = 1; month <= 12; month++ ) { printf("%s, %d", month_name[month], year); printf("\n\nSun Mon Tue Wed Thu Fri Sat\n" ); /*For printing the first date on correct position, use some space to move the cursor on the correct location to print first date under proper day name */ for ( day = 1; day <= 1 + daycode * 5; day++ ) { printf(" "); } /* For loop from day 1 to last day of month,for printing all the dates for one month*/ for ( day = 1; day <= month_days[month]; day++ ) { printf("%2d", day ); /* Checking if day before Saturday, otherwise start next line from Sunday.*/ if ( ( day + daycode ) % 7 > 0 ) printf(" " ); else printf("\n " ); } /* Here setting position for next month, according to the daycode*/ daycode = ( daycode + month_days[month] ) % 7; } } int main(void) { int year, daycode, leapyear; printf("Please Enter a Four Digit Year,{Example} 2020 : "); scanf("%d", &year); daycode = get_first_daycode(year); set_feb_days(year); print_calendar(year, daycode); printf("\n"); return 0; }
Declaration of Two Global Arrays first of all, this C language Calendar program defines two global arrays. The first global array is month_days with total 13 elements but for simplicity we are using the index 1 to 12 for storing days in January to December. Similarly, the second global array named month_name stores the names of the months from January to December. We do not use the first location for the same reason as we mentioned above. int month_days[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; char *month_name[]= { " ", "\n\n\nJanuary", "\n\n\nFebruary", "\n\n\nMarch", "\n\n\nApril", "\n\n\nMay", "\n\n\nJune", "\n\n\nJuly", "\n\n\nAugust", "\n\n\nSeptember", "\n\n\nOctober", "\n\n\nNovember", "\n\n\nDecember" }; /* This functions returns daycode of the first day of the year. if daycode is 1, first day is Monday */ int get_first_daycode(int year) { int daycode; int x, y, z; x = (year - 1) / 4; y = (year - 1) / 100; z = (year - 1) / 400; daycode = (year + x - y + z) %7; return daycode; } This function void set_feb_days(int year), checks for the leap year and sets the number of days in Februry accordingly as 29 or 28. void set_feb_days(int year) { if(year% 4 == 0 && year%100 != 0 || year%400 == 0) month_days[2] = 29; else month_days[2] = 28; } The following function prints the calendar for the 12 months of the given year. void print_calendar(int year, int daycode) { int month, day; for ( month = 1; month <= 12; month++ ) { printf("%s, %d", month_name[month], year); printf("\n\nSun Mon Tue Wed Thu Fri Sat\n" ); /*For printing the first date on correct position, use some space to move the cursor on the correct location to print first date under proper day name */ for ( day = 1; day <= 1 + daycode * 5; day++ ) { printf(" "); } /* For loop from day 1 to last day of month,for printing all the dates for one month*/ for ( day = 1; day <= month_days[month]; day++ ) { printf("%2d", day ); /* Checking if day before Saturday, otherwise start next line from Sunday.*/ if ( ( day + daycode ) % 7 > 0 ) printf(" " ); else printf("\n " ); } /* Here setting position for next month, according to the daycode*/ daycode = ( daycode + month_days[month] ) % 7; } } The main() function inputs the year in four digits to print the calendar and passes it to the three functions defined in this program to print the required calendar. int main(void) { int year, daycode, leapyear; printf("Please Enter a Four Digit Year,{Example} 2020 : "); scanf("%d", &year); daycode = get_first_daycode(year); set_feb_days(year); print_calendar(year, daycode); printf("\n"); return 0; }
Please Enter a Four Digit Year,{Example} 2020 : 2020 January, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 February, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 March, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 April, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 May, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 June, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 July, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 August, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 September, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 October, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 November, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 December, 2020 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 Press Enter to return to Quincy…
Display Yearly Calendar C Program
C Programs on Files:
Therefore, we study today, how to display a calendar in C Programming Language. Here are some File Programs in C Language, you will love to read:
- C File Program to Save Records in a Binary File
- C Program to Read Records From Binary File
- C Program to Append / Add More Records in Binary File
- C Program to Search a Record in Binary File C Programming
- C Program to Search a Record by Name in Binary File
- C Program to Search Multiple Records of Given Name in Binary File