Check Palindrome String C Program – Input a string and check whether this string entered by the user is a Palindrome or Not.
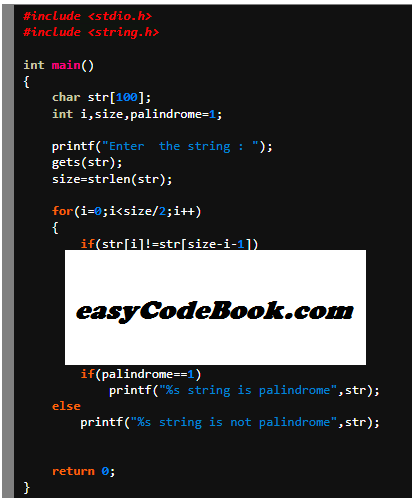
Source Code
#include <stdio.h> #include <string.h> int main() { char str[100]; int i,size,palindrome=1; printf("Enter the string : "); gets(str); size=strlen(str); for(i=0;i<size/2;i++) { if(str[i]!=str[size-i-1]) { palindrome=0; break; } } if(palindrome==1) printf("%s string is palindrome",str); else printf("%s string is not palindrome",str); return 0; }
Detailed Explanation of Source Code
Let’s go through the given C program line by line and provide an explanation for each line:
These are preprocessor directives that include the necessary header files for the program. <stdio.h>
is required for input/output operations, and <string.h>
is needed for string manipulation functions.
int main()
{
char str[100];
int i, size, palindrome = 1;
This is the main function of the program. It declares a character array str
of size 100 to store the input string. It also declares integer variables i
, size
, and palindrome
, where palindrome
is initialised to 1.
printf("Enter the string: ");
gets(str);
This line prompts the user to enter a string and stores it in the str
array using the gets()
function. Note that gets()
is generally discouraged to use due to potential buffer overflow issues. A safer alternative would be to use fgets()
.
size = strlen(str);
This line calculates the length of the string entered by the user using the strlen()
function and assigns it to the size
variable.
Main Loop To Check for Palindrome String
for (i = 0; i < size / 2; i++)
{
if (str[i] != str[size - i - 1])
{
palindrome = 0;
break;
}
}
This for
loop checks if the string is a palindrome. It iterates from the beginning of the string (i = 0
) up to half its length (size / 2
). It compares the character at position i
with the character at position size - i - 1
(which is the corresponding character from the end of the string). If there is any mismatch, the palindrome
variable is set to 0, indicating that the string is not a palindrome. The loop is also terminated using break
.
if (palindrome == 1)
printf("%s string is palindrome", str);
else
printf("%s string is not palindrome", str);
This if-else
statement checks the value of the palindrome
variable. If it is 1, it means the string is a palindrome, so the corresponding message is printed. Otherwise, if palindrome
is 0, the string is not a palindrome, and the respective message is printed.
return 0;
}
This statement terminates the main()
function and returns 0, indicating successful execution of the program.
Output
Enter the string : mom
mom string is palindrome
——————————–
Output 2:
Enter the string : father
father string is not palindrome
Palindrome string checking Program – Summary: This C program checks whether a given string is a palindrome or not. It takes a string as input, compares the characters from the beginning and end of the string, and determines if it is a palindrome. The program then outputs whether the string is a palindrome or not.