Count Words in String C Program – Write down a C program to input a string and find the number of words in it.
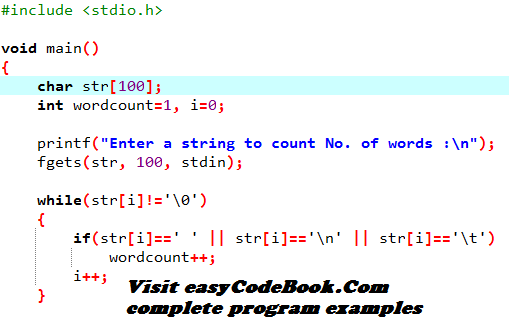
Count words in string C Programming
Source Code
#include <stdio.h> void main() { char str[100]; int wordcount=1, i=0; printf("Enter a string to count No. of words :\n"); fgets(str, 100, stdin); while(str[i]!='\0') { if(str[i]==' ' || str[i]=='\n' || str[i]=='\t') wordcount++; i++; } printf("Total number of words in the string: %d", wordcount-1); }
Output
Enter a string to count No. of words : Easy Code Book Total number of words in the string: 3
Line by Line Explanation of Source Code
Let’s go through the code step by step and explain each part along with a sample output using the string “Hello World of C Programming”:
Explanation:
- The code begins with the inclusion of the standard input/output library
stdio.h
, which provides functions for input and output operations. - The
main
function is defined, which is the entry point of the program. - Inside the
main
function, several variables are declared.char str[100]
is an array of characters that will hold the input string.int wordcount
is an integer variable that will store the count of words in the string.int i
is an integer variable used as an index for iterating through the characters of the string.
- The
printf
function is used to display the prompt message to the user: “Enter a string to count No. of words :”. - The
fgets
function is used to read a line of input from the user. It takes three arguments:str
is the destination buffer where the input will be stored.100
is the maximum number of characters to be read (including the null character).stdin
is the standard input stream from which the input is read (in this case, it’s the keyboard).
Sample output:
Enter a string to count No. of words :
Hello World of C Programming
- The program enters a
while
loop that iterates over each character in the string until it encounters the null character'\0'
, which marks the end of the string. - Inside the loop, there is an
if
condition that checks if the current characterstr[i]
is a space' '
, newline'\n'
, or tab'\t'
. If any of these characters are found, it means a word boundary has been reached, so thewordcount
is incremented. - After the
if
condition, the index variablei
is incremented to move to the next character in the string. - Once the loop finishes, the total number of words is calculated by subtracting 1 from
wordcount
(since we initially set it to 1). This is done to account for the fact that the last word doesn’t have a word boundary character after it. - Finally, the
printf
function is used to display the total number of words in the string. The value ofwordcount-1
is substituted in the format string, and the result is printed.
Sample output:
Total number of words in the string: 5
In the given example, the output is 5, which represents the number of words in the string “Hello World of C Programming.”