Count Digits in String C Program – Input a string containing both digits and alphabets. This program in C language will display the count of digits in the given string.
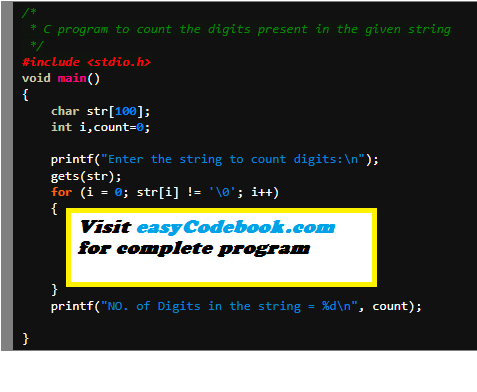
Source Code
/* * C program to count the digits present in the given string */ #include <stdio.h> void main() { char str[100]; int i,count=0; printf("Enter the string to count digits:\n"); gets(str); for (i = 0; str[i] != '\0'; i++) { if ((str[i] >= '0') && (str[i] <= '9')) { count++; } } printf("NO. of Digits in the string = %d\n", count); }
Output
Enter the string to count digits: abc123xyz NO. of Digits in the string = 3
How This Program Works
The given C program is designed to count the number of digits present in a given string. Let’s walk through the logic step by step:
- The program starts by including the standard input/output library
stdio.h
. - The
main
function is defined, serving as the entry point of the program. - Inside the
main
function, several variables are declared:char str[100]
is an array of characters that will hold the input string.int i
is an integer variable used as an index for iterating through the characters of the string.int count
is an integer variable initialized to zero, which will store the count of digits in the string.
- The
printf
function is used to display a prompt message asking the user to enter a string to count digits. - The
gets
function is used to read a line of input from the user and store it in thestr
array.
- A
for
loop is used to iterate through each character of the string until the null character'\0'
is encountered, indicating the end of the string. - Inside the loop, an
if
condition checks if the current characterstr[i]
is between'0'
and'9'
, which represent the ASCII values of digits 0 to 9. If the condition is true, it means the current character is a digit, so thecount
variable is incremented. - After each iteration, the index
i
is incremented to move to the next character in the string.
- Finally, the
printf
function is used to display the count of digits in the string. The value of thecount
variable is substituted in the format string, and the result is printed.
In summary, the program reads a string from the user and counts the number of digits present in the string. It iterates through each character of the string and increments the count whenever a digit is found. The final count is then displayed as output.