Q: Write a C Program to Find Smallest Number in Array
The Basic Logic of Smallest Number in Array Program
- First of all the user will input any 5 numbers in array.
- The program assumes that the first number is minimum number
- min = numArray[0];
- This C program uses a for loop to get each number in the given array and compare it with current minimum number. If the current item of array is less than current minimum number, then assign current array item to min
- if( numArray[i] < min)
- min = numArray[i];
- After termination of loop the minimum number will be in min variable.
- Display the value of min variable
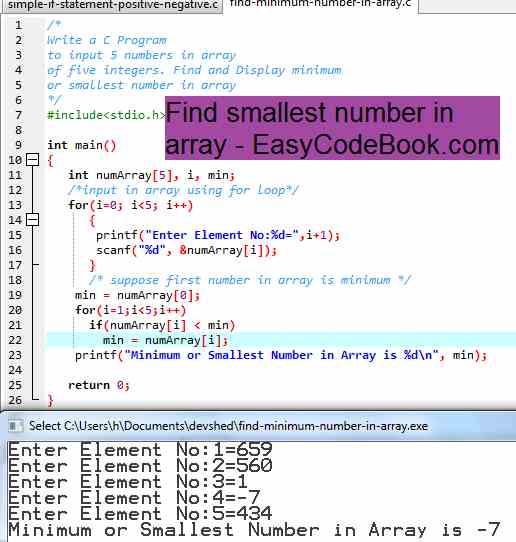
The Source Code for c program to show minimum number in array is as follows:
/* Write a C Program to input 5 numbers in array of five integers. Find and Display minimum or smallest number in array */ #include<stdio.h> int main() { int numArray[5], i, min; /*input in array using for loop*/ for(i=0; i<5; i++) { printf("Enter Element No:%d=",i+1); scanf("%d", &numArray[i]); } /* suppose first number in array is minimum */ min = numArray[0]; for(i=1;i<5;i++) if(numArray[i] < min) min = numArray[i]; printf("Minimum or Smallest Number in Array is %d\n", min); return 0; }