Understanding C Pointers – will give you a deep understanding of pointer concept in C programming language.
What is a Pointer in C?
A Pointer is a special variable that can hold the memory address of another variable. We will use the reference operator ( & ) to store memory address in a pointer variable.
Explanation
Every variable has a name, value and a memory address in computer memory. For example, let us declare / initialise an integer variable “num” as shown below:
int num=100;
Let us understand this concept with a diagram:
As shown in above picture, the variable num has the value 100 and its address in memory is 000000000022FE4C. The point to understand is that we can use a pointer variable that holds this address 000000000022FE4C. The address of the variable is stored in pointer variable with the help of address of operator &.
C Program and Output To Explain Memory Address of Variable
#include <stdio.h> int main() { /* Initialize an integer varible num with value 100 */ int num=100; /* Here we display value and address of num variable */ printf("The value of num variable is: %d",num); printf("\nThe address of num variable is: %p",&num); return 0; }
Output
The value of num variable is: 100
The address of num variable is: 000000000022FE4C
Pointer Declaration in C
A pointer variable in C Language is declared with the following Syntax:
type *identifier;
Here type is the data type of the variable to which pointer will point. * operator declares a pointer and identifier is the name of the pointer variable.
Example:
int *ptr;
Here ptr is an integer pointer. It means that ptr can hold the address of an integer variable. Similarly, we may declare the pointer variables to hold the address of the variables of other data type like char, long and float variables etc.
Operators Used With Pointers in C Language
Address Of Operator ( & )
The address of operator & is used before the variable name to get the address of that variable. We may store the address of a variable in a pointer using Address of ( & ) operator.
Example:
int num = 100;
int *ptr;
ptr = # /*store address of num in ptr*/
int *ptr;
ptr = # /*store address of num in ptr*/
Deference Operator ( * )
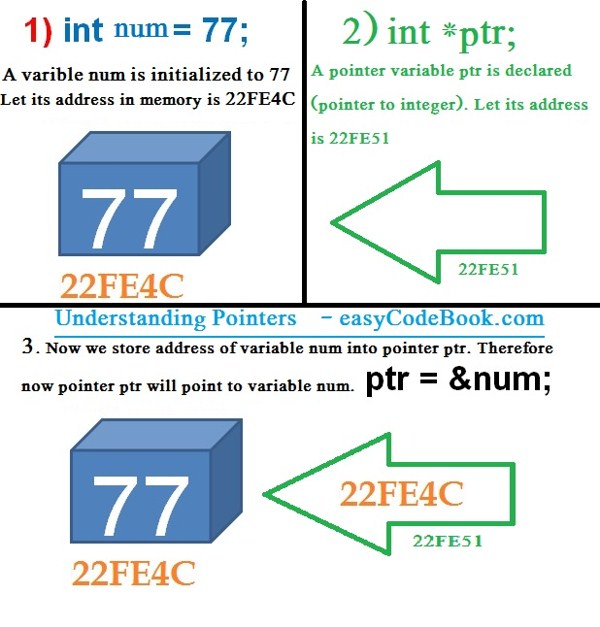
Example C Program To Declare and Use Pointer Variable
#include <stdio.h> int main() { /* Initialize an integer varible num with value 100 */ int num=100; /* Declare an pointer variable pointer to int */ int *ptr; /* Store address of num variable in ptr */ ptr = # /* Here we display value and address of num variable */ printf("The value of num variable is: %d",num); printf("\nThe address of num variable is: %p",&num); printf("\n------------------------------\n"); /*Use derefernce operator before ptr to access value*/ printf("The value of num variable using Pointer is: %d",*ptr); /* show value of ptr (address of num)*/ printf("The address of num variable in Pointer ptr is: %p",ptr); return 0; }
Output
The value of num variable is: 100 The address of num variable is: 000000000022FE44 ------------------------------ The value of num variable using Pointer is: 100 The address of num variable in Pointer ptr is: 000000000022FE44
You may like to learn Write a C Program to Add Two Numbers Using Pointers.