PyQt Program To Add Remove Clear Items From List –
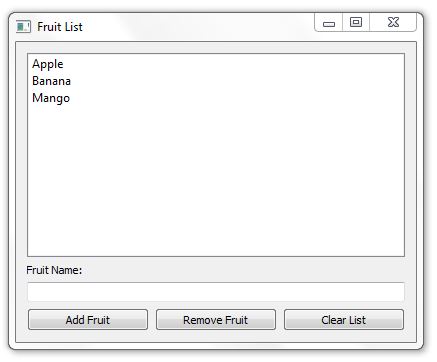
Source Code
import sys from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QHBoxLayout, QPushButton, QLineEdit, QListWidget, QLabel class FruitListApp(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle('Fruit List') self.setGeometry(100, 100, 400, 300) self.fruit_list = QListWidget(self) self.add_button = QPushButton('Add Fruit', self) self.remove_button = QPushButton('Remove Fruit', self) self.clear_button = QPushButton('Clear List', self) self.name_input = QLineEdit(self) self.name_label = QLabel('Fruit Name:', self) self.add_button.clicked.connect(self.addFruit) self.remove_button.clicked.connect(self.removeFruit) self.clear_button.clicked.connect(self.clearList) vbox = QVBoxLayout() hbox = QHBoxLayout() vbox.addWidget(self.fruit_list) vbox.addWidget(self.name_label) vbox.addWidget(self.name_input) hbox.addWidget(self.add_button) hbox.addWidget(self.remove_button) hbox.addWidget(self.clear_button) vbox.addLayout(hbox) self.setLayout(vbox) def addFruit(self): fruit_name = self.name_input.text() if fruit_name: self.fruit_list.addItem(fruit_name) self.name_input.clear() def removeFruit(self): selected_item = self.fruit_list.currentItem() if selected_item: self.fruit_list.takeItem(self.fruit_list.row(selected_item)) def clearList(self): self.fruit_list.clear() def main(): app = QApplication(sys.argv) ex = FruitListApp() ex.show() sys.exit(app.exec_()) if __name__ == '__main__': main()
In the PyQt program provided, a QListWidget
widget is used to display and manage a list of items (in this case, the names of fruits). Here, I’ll explain the QListWidget
methods and properties that are used in the application:
QListWidget
:self.fruit_list = QListWidget(self)
: This line creates an instance of theQListWidget
class, which is used to display the list of fruits. It is added to the main window (self
) as a child widget.
QListWidget
Methods:addItem(text)
: TheaddItem
method is used to add an item to the list. In this case, it’s used to add the names of fruits to theQListWidget
when the “Add Fruit” button is clicked.takeItem(row)
: ThetakeItem
method is used to remove an item from the list. It takes the row index of the item to be removed as an argument. In this application, it’s used when the “Remove Fruit” button is clicked to remove the selected fruit from the list.clear()
: Theclear
method removes all items from the list. It’s used when the “Clear List” button is clicked to clear all the fruit names from theQListWidget
.
QListWidget
Properties:currentItem()
: This property returns the currently selected item in the list. It’s used to determine which item is selected when the “Remove Fruit” button is clicked.row(item)
: This property returns the row index of the given item in the list. It’s used to identify the row of the selected item when removing it.addItem(text)
,takeItem(row)
, andclear()
methods are used in conjunction with these properties to add, remove, and clear items in theQListWidget
.
The QListWidget
widget provides a convenient way to work with lists of items in PyQt applications. You can add, remove, and manipulate items as needed, as demonstrated in this example.
- Common PyQt GUI Widgets
- PyQt Introduction and Features
- Basic Structure of PyQt GUI Program
- Python PyQt Convert Kilograms To Pounds
- Python PyQt Convert Pounds To Kilograms
- Python PyQt Find Factorial
- Python PyQt Add Two Numbers