Python PyQt Find Factorial – To create a PyQt GUI program that calculates the factorial of a number, you can use a similar approach as in the previous example. Here’s a basic PyQt program to calculate the factorial of a number entered by the user:
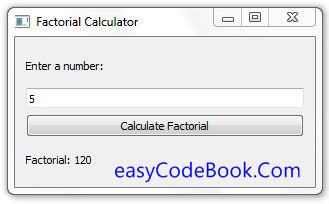
Python PyQt Find Factorial
Source Code
import sys from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel, QLineEdit, QPushButton class FactorialCalculator(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle('Factorial Calculator') self.setGeometry(100, 100, 300, 150) # Create a vertical layout layout = QVBoxLayout() # Create input field for the number self.num_label = QLabel('Enter a number:') self.num_input = QLineEdit() # Create a label to display the result self.result_label = QLabel('Factorial:') # Create a button to calculate factorial self.calculate_button = QPushButton('Calculate Factorial') # Connect the button click event to the factorial calculation function self.calculate_button.clicked.connect(self.calculateFactorial) # Add widgets to the layout layout.addWidget(self.num_label) layout.addWidget(self.num_input) layout.addWidget(self.calculate_button) layout.addWidget(self.result_label) # Set the layout for the main window self.setLayout(layout) def calculateFactorial(self): try: # Get the number from the input field num = int(self.num_input.text()) # Check if the number is negative if num < 0: self.result_label.setText('Factorial is not defined for negative numbers') else: # Calculate the factorial result = 1 for i in range(1, num + 1): result *= i # Display the result self.result_label.setText(f'Factorial: {result}') except ValueError: # Handle invalid input (e.g., non-integer input) self.result_label.setText('Invalid input') def main(): app = QApplication(sys.argv) window = FactorialCalculator() window.show() sys.exit(app.exec_()) if __name__ == '__main__': main()
This code creates a PyQt application with a window containing an input field for the user to enter a number, a button to calculate the factorial, and a label to display the result. When the user enters a number and clicks the “Calculate Factorial” button, the factorial of the number is calculated and displayed in the label.
Make sure you have PyQt5 installed (pip install PyQt5) before running this code.