Common GUI Widgets of PyQt – PyQt5 is a Python library for creating graphical user interfaces (GUIs) with the Qt framework.
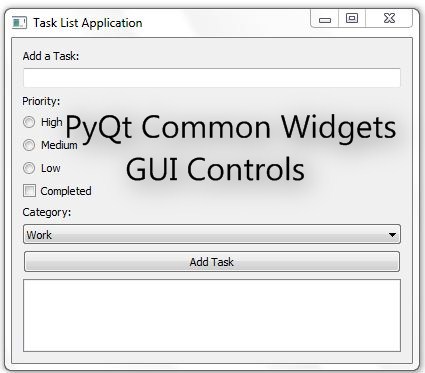
It provides a wide range of widgets that you can use to build your GUI applications. Here, I’ll explain some common widgets along with their properties and methods:
- QWidget:
- Introduction:
QWidget
is the base class for all user interface objects in PyQt5. It represents a rectangular area on the screen and can be used to create custom widgets or serve as the main application window. - Uses: It is the foundation for building GUI applications, and you can add other widgets as child components to it.
- Introduction:
- QLabel:
- Introduction:
QLabel
is used to display text or images in a PyQt5 application. It is a non-editable text or image container. - Uses: Displaying static text, captions, or images within your GUI.
- Introduction:
- QLineEdit:
- Introduction:
QLineEdit
is a single-line text input field that allows users to enter text. - Uses: Taking user input for various purposes like searching, data entry, or configuration settings.
- Introduction:
- QPushButton:
- Introduction:
QPushButton
is a clickable button that performs an action when clicked. - Uses: Triggering actions, executing functions, and handling user interactions such as submitting forms or starting processes.
- Introduction:
- QRadioButton:
- Introduction:
QRadioButton
is a selectable option in a group of mutually exclusive options, represented as radio buttons. - Uses: Creating groups of options where users can select only one choice, like choosing a mode or preference.
- Introduction:
- QCheckBox:
- Introduction:
QCheckBox
is a widget that allows users to toggle between two states (checked or unchecked). - Uses: Enabling/disabling features, options, or settings within an application.
- Introduction:
- QComboBox:
- Introduction:
QComboBox
is a drop-down list of items from which users can select one option. - Uses: Providing a list of choices when space is limited or when you want to allow users to select from predefined options.
- Introduction:
- QListWidget:
- Introduction:
QListWidget
is a widget that displays a list of items. Each item can have associated text or icons. - Uses: Displaying and selecting items from a list, creating file explorers, or building interfaces with multiple selectable items.
- Introduction:
Properties and Methods of Common Widgets
- QWidget:
- Properties:
geometry
: Defines the size and position of the widget.visible
: Indicates whether the widget is visible or not.
- Methods:
show()
: Displays the widget.hide()
: Hides the widget.
- Properties:
- QLabel:
- Properties:
text
: Sets or retrieves the text displayed by the label.
- Methods:
setText()
: Sets the text of the label.text()
: Retrieves the text of the label.
- Properties:
- QLineEdit:
- Properties:
text
: Gets or sets the text entered in the line edit.
- Methods:
setText()
: Sets the text in the line edit.text()
: Retrieves the text from the line edit.
- Properties:
- QPushButton:
- Properties:
text
: Sets or retrieves the text on the button.
- Methods:
setText()
: Sets the text on the button.text()
: Retrieves the text from the button.
- Properties:
- QRadioButton:
- Properties:
text
: Sets or retrieves the text associated with the radio button.isChecked
: Indicates whether the radio button is checked.
- Methods:
setText()
: Sets the text for the radio button.text()
: Retrieves the text of the radio button.isChecked()
: Checks if the radio button is selected.setChecked()
: Sets the radio button’s checked state.
- Properties:
- QCheckBox:
- Properties:
text
: Sets or retrieves the text associated with the checkbox.isChecked
: Indicates whether the checkbox is checked.
- Methods:
setText()
: Sets the text for the checkbox.text()
: Retrieves the text of the checkbox.isChecked()
: Checks if the checkbox is selected.setChecked()
: Sets the checkbox’s checked state.
- Properties:
- QComboBox:
- Properties:
currentText
: Retrieves the currently selected text.
- Methods:
addItem()
: Adds an item to the combo box.removeItem()
: Removes an item from the combo box.currentIndex()
: Retrieves the index of the currently selected item.currentText()
: Retrieves the text of the currently selected item.
- Properties:
- QListWidget:
- Properties:
currentItem
: Retrieves the currently selected item.
- Methods:
addItem()
: Adds an item to the list.takeItem()
: Removes an item from the list.currentItem()
: Retrieves the currently selected item.
- Properties:
These widgets are essential building blocks for creating a wide range of GUI applications in PyQt5. By combining and customizing them, you can design user-friendly and interactive interfaces for your Python applications. Each widget has specific properties and methods that allow you to control and manipulate its behavior to meet your application’s requirements.
You may also like to Read more on PyQt Tutorials and GUI Program Examples:
- PyQt Introduction and Features
- Basic Structure of PyQt GUI Program
- Python PyQt Convert Kilograms To Pounds
- Python PyQt Convert Pounds To Kilograms
- Python PyQt Find Factorial
- Python PyQt Add Two Numbers