PyQt Four Function Calculator – To create a PyQt program with three QLineEdit
widgets for input, labels, six buttons for performing mathematical operations (Add, Subtract, Multiply, Divide, Clear, Exit), and a third QLineEdit
widget for displaying the result, you can use the following code:
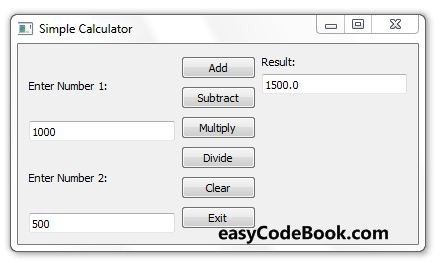
PyQt Four Function Calculator
import sys from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QHBoxLayout, QLabel, QLineEdit, QPushButton class CalculatorApp(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle('Simple Calculator') self.setGeometry(100, 100, 400, 200) self.num1_label = QLabel('Enter Number 1:') self.num1_edit = QLineEdit() self.num2_label = QLabel('Enter Number 2:') self.num2_edit = QLineEdit() self.result_label = QLabel('Result:') self.result_edit = QLineEdit() self.result_edit.setReadOnly(True) self.add_button = QPushButton('Add') self.subtract_button = QPushButton('Subtract') self.multiply_button = QPushButton('Multiply') self.divide_button = QPushButton('Divide') self.clear_button = QPushButton('Clear') self.exit_button = QPushButton('Exit') self.add_button.clicked.connect(self.add) self.subtract_button.clicked.connect(self.subtract) self.multiply_button.clicked.connect(self.multiply) self.divide_button.clicked.connect(self.divide) self.clear_button.clicked.connect(self.clear) self.exit_button.clicked.connect(self.close) # Layout input_layout = QVBoxLayout() input_layout.addWidget(self.num1_label) input_layout.addWidget(self.num1_edit) input_layout.addWidget(self.num2_label) input_layout.addWidget(self.num2_edit) button_layout = QVBoxLayout() button_layout.addWidget(self.add_button) button_layout.addWidget(self.subtract_button) button_layout.addWidget(self.multiply_button) button_layout.addWidget(self.divide_button) button_layout.addWidget(self.clear_button) button_layout.addWidget(self.exit_button) result_layout = QVBoxLayout() result_layout.addWidget(self.result_label) result_layout.addWidget(self.result_edit) result_layout.addStretch(1) # Add this line to stretch the result_edit widget main_layout = QHBoxLayout() main_layout.addLayout(input_layout) main_layout.addLayout(button_layout) main_layout.addLayout(result_layout) self.setLayout(main_layout) def add(self): try: num1 = float(self.num1_edit.text()) num2 = float(self.num2_edit.text()) result = num1 + num2 self.result_edit.setText(str(result)) except ValueError: self.result_edit.setText('Invalid Input') def subtract(self): try: num1 = float(self.num1_edit.text()) num2 = float(self.num2_edit.text()) result = num1 - num2 self.result_edit.setText(str(result)) except ValueError: self.result_edit.setText('Invalid Input') def multiply(self): try: num1 = float(self.num1_edit.text()) num2 = float(self.num2_edit.text()) result = num1 * num2 self.result_edit.setText(str(result)) except ValueError: self.result_edit.setText('Invalid Input') def divide(self): try: num1 = float(self.num1_edit.text()) num2 = float(self.num2_edit.text()) if num2 == 0: self.result_edit.setText('Division by zero') else: result = num1 / num2 self.result_edit.setText(str(result)) except ValueError: self.result_edit.setText('Invalid Input') def clear(self): self.num1_edit.clear() self.num2_edit.clear() self.result_edit.clear() if __name__ == '__main__': app = QApplication(sys.argv) window = CalculatorApp() window.show() sys.exit(app.exec_())
This code creates a simple calculator application using PyQt. It has three input fields (for two numbers and result display), labels, and buttons for performing basic arithmetic operations. When you click the operation buttons, it calculates the result and displays it in the third input field.
- PyQt Program To Add Remove Clear Items From List
- Common PyQt GUI Widgets
- PyQt Introduction and Features
- Basic Structure of PyQt GUI Program
- Python PyQt Convert Kilograms To Pounds
- Python PyQt Convert Pounds To Kilograms
- Python PyQt Find Factorial
- Python PyQt Add Two Numbers