Programming Mouse Buttons in C Sharp – This is a windows forms application that will check whether the user has clicked a left mouse button or a right mouse button. It will response accordingly. Let us suppose that we will change the form’s back color to RED when user clicks left button and GREEN when the user presses Right button.
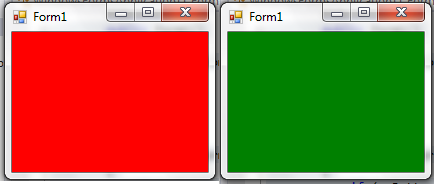
Programming Mouse Buttons in C Sharp
Source Code
using System; using System.Drawing; using System.Windows.Forms; namespace WindowsFormsApplication11 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_MouseClick(object sender, MouseEventArgs e) { if (e.Button == MouseButtons.Left) this.BackColor = Color.Red; else if (e.Button == MouseButtons.Right) this.BackColor = Color.Green; } } }
Explanation
The main part of this example is the Form1_MouseClick event handler method. This method is automatically generated when you double-click on the form in the Visual Studio designer, associating it with the MouseClick event of the form.
The sender parameter of the event handler represents the object that triggered the event, in this case, the form itself.
The e parameter of the event handler contains information about the event, including the properties of the mouse click. The MouseButtons enumeration is used to specify which mouse button was clicked.
Inside the event handler, there’s an if statement that checks the value of e.Button to determine which mouse button was clicked. If the left mouse button was clicked, the background color of the form is changed to red. If the right mouse button was clicked, the background color is changed to green.
In summary, this code creates a Windows Forms application with a form (window) that changes its background color based on the mouse button clicked. If the left mouse button is clicked, the background color becomes red, and if the right mouse button is clicked, the background color becomes green. This demonstrates the basic concept of handling mouse click events in a graphical user interface.
In C#, you can determine which mouse button was clicked by examining the Button
property of the MouseEventArgs
object provided by the mouse-related events. This property gives you information about the button that triggered the event. The MouseButtons
enumeration defines constants for different mouse buttons, which you can use to compare against the Button
property.
Here are some of the common values defined in the MouseButtons
enumeration:
MouseButtons.Left
: Represents the left mouse button.MouseButtons.Right
: Represents the right mouse button.MouseButtons.Middle
: Represents the middle mouse button (often associated with the scroll wheel).MouseButtons.XButton1
: Represents the first extra mouse button.MouseButtons.XButton2
: Represents the second extra mouse button.
You can use these constants to handle mouse events and perform different actions based on the buttons that were pressed or released.