Designing Digital Clock in Visual Programming – Create a Windows forms application to design a digital clock using C# in visual Studio.
Timer Control
Timer control is used to executes a code block repeatedly at a specified interval. For example, to display time every second or backing up a folder every 10 minutes or writing to a log file
every second. The method that needs to be executed is placed inside the timer Tick event.
Windows Forms has a Timer control that can be dropped to a Form and set its properties. A Timer control does not have a visual representation and works as a component in the background.
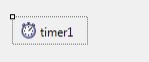
Interval property
It is used to set time interval in milliseconds after which the Tick event is generated.
Enabled Property
Enabled property can be set to true or false. It tells whether a timer is running or not. By default the Enabled property of Timer Control is False. So before running the program we have to set the Enabled property is True , then only the Timer Control starts its function.
Tick Event
Tick event of timer control occurs after the specified number of
milliseconds in interval property. We can write here any code to be
executed repeatedly after a specified time interval.
C# program to display Digital Clock using timer control
1. Create a new Windows Forms Application project. Name the project as DigitalClock.
2. Then go to the form’s design view. Add a Label and a Timer control. Our form will look such as below:
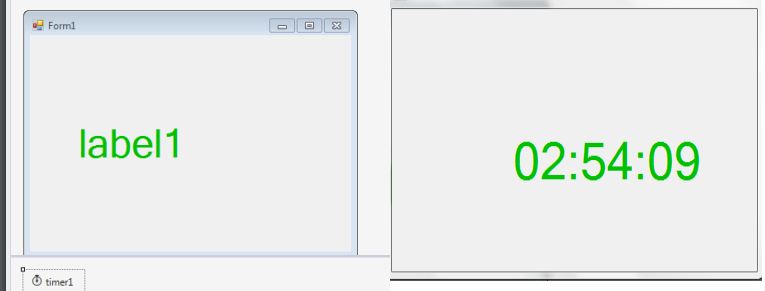
How to design a digital clock in C Sharp Visual Programming
It contains a label at the center of the form and a timer control. So drag a label and a timer from the toolbox onto the form. Change the color of the label and increase it’s text size(font size) and fore color property to green.
3. Double click on timer control and write code in your timer_tick event for the timer control.
private void timer1_Tick(object sender, EventArgs e)
{
label1.Text = DateTime.Now.ToString(“HH:mm:ss”);
}
Here DateTime represents an instance of a time of a day and Now represents the current date and time of your computer. HH:mm:ss represents the time format as you wish to display it in this format. HH means hours in 24 Hour format, mm means minutes ss means seconds.
4. Right-click on the timer go to properties. Set Enabled property to True. Set the Interval property to 1000.
5. Start (execute) the project to display digital clock.
Source Code: Designing Digital Clock in Visual Programming
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace DigitalControl { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void timer1_Tick(object sender, EventArgs e) { label1.Text = DateTime.Now.ToString("HH:mm:ss"); } } }
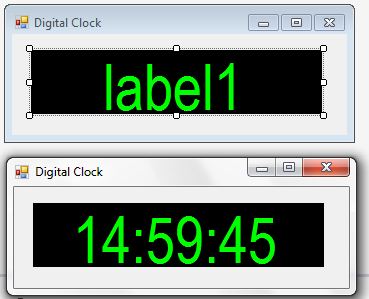
Designing Digital Clock in Visual Programming
Explanation of the Digital Clock Application
- The program is defined in the
DigitalControl
namespace and contains a partial class namedForm1
, which represents the main form of the application. - The
Form1
constructor (public Form1()
) initializes the components of the form. This constructor is responsible for setting up the GUI components and their initial properties. - The
timer1_Tick
method is an event handler that executes at specified intervals. It’s triggered by theTick
event of thetimer1
component. - Inside the
timer1_Tick
method:- The method updates the
Text
property oflabel1
with the current time in “HH:mm:ss” format.DateTime.Now
gets the current date and time, and.ToString("HH:mm:ss")
formats the time to display hours, minutes, and seconds.
- The method updates the
- The program uses a timer (
timer1
) to update the time display at regular intervals. TheTick
event of the timer is wired to thetimer1_Tick
event handler. - The timer’s interval (the time between ticks) is not specified in this code snippet. It would have been set in the properties window of the form designer when creating the application.
When this program is run, it will display a Windows Form with a label (likely named label1
by default). The label will continuously show the current time in the format “HH:mm:ss” and update at the interval specified by the timer. This example demonstrates how to create a simple digital clock display using Windows Forms and timers in C#.