Employee Database Save Find Record – C Sharp Database Application – Windows Forms Application in C Sharp / Visual Studio.
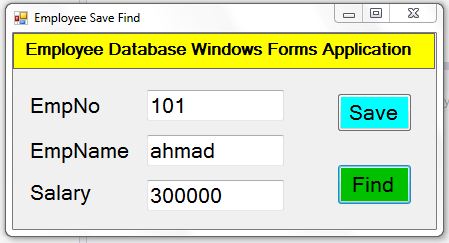
Source Code
using System;
using System.Windows.Forms;
using System.Data.OleDb;
namespace EmployeeSaveFind
{
public partial class Employee : Form
{
OleDbConnection connection;
public Employee()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
string constring = "Provider=Microsoft.ACE.Oledb.12.0;Data Source=EmployeeDB.accdb;";
connection = new OleDbConnection(constring);
connection.Open();
}
private void button1_Click(object sender, EventArgs e)
{
if (txtEmpNo.Text == "" || txtEmpName.Text == "" || txtSalary.Text == "")
{
MessageBox.Show("Enter values in all text boxes");
return;
}
string query;
query = "INSERT INTO emp VALUES(" + txtEmpNo.Text + "," + "'" + txtEmpName.Text + "'" + "," + txtSalary.Text + ")";
OleDbCommand command = new OleDbCommand(query, connection);
try
{
command.ExecuteNonQuery();
MessageBox.Show("Record Saved");
txtEmpNo.Clear();
txtEmpName.Clear();
txtSalary.Clear();
txtEmpNo.Focus();
}
catch (Exception exp)
{
MessageBox.Show(exp.Message);
}
}
private void button2_Click(object sender, EventArgs e)
{
OleDbDataReader reader;
string query;
query = "Select * from Emp where EmpNo=" + txtEmpNo.Text;
OleDbCommand command = new OleDbCommand(query, connection);
reader = command.ExecuteReader();
if (reader.Read())
{
txtEmpName.Text = reader[1].ToString();
txtSalary.Text = reader[2].ToString();
}
else
{
MessageBox.Show("Record Not Found");
}
reader.Close();
}
}
}
Explanation
This C# program is a Windows Forms application that allows users to save and find employee records in a database. The database used is an MS Access database named “EmployeeDB.accdb”. Let’s break down the key components and explain the functionality step by step:
Explanation of the main points:
- Namespace and Class: The program is defined in the
EmployeeSaveFind
namespace and contains a partial class namedEmployee
, which is a Windows Forms class. - Constructor and Form Load: The
Employee
class’s constructor initializes the form components, and theForm1_Load
method is triggered when the form loads. It establishes a connection to the database using an OleDb connection string. - Save Record (button1_Click): When the “Save Record” button is clicked, the program checks if all text boxes (
txtEmpNo
,txtEmpName
, andtxtSalary
) have values. If not, a message is displayed. Otherwise, an SQL query is constructed to insert a new record into theemp
table. TheExecuteNonQuery
method is used to execute the query, and success or error messages are shown accordingly. - Find Record (button2_Click): When the “Find Record” button is clicked, an SQL query is constructed to retrieve a record from the
emp
table based on the provided employee number (txtEmpNo
). TheExecuteReader
method is used to execute the query, and if a record is found, the employee name and salary are displayed in the respective text boxes. If no record is found, a message is shown. - Closing Connections: The
OleDbDataReader
(reader
) is explicitly closed after use to release database resources.