Insert Phone Record into Table Visual Programming – Consider a table Phone in database named Mobile. Write C Sharp Code to INSERT data INTO database after creating appropriate form using this table and database connection.
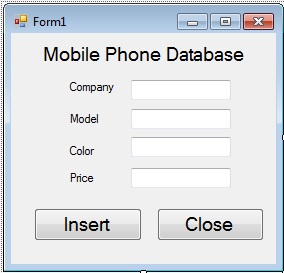
Insert Phone Record into Table Visual Programming
Source Code
using System; using System.Data.OleDb; using System.Windows.Forms; namespace MobileDB { public partial class Form1 : Form { OleDbConnection connection; public Form1() { InitializeComponent(); } //CODE for INSERT button private void button2_Click(object sender, EventArgs e) { if (textBox1.Text == "" || textBox2.Text == "" || textBox3.Text == "" || textBox4.Text == "") { MessageBox.Show("Enter values in all text boxes"); return; } string query; query = "INSERT INTO Phone VALUES("+ "'" + textBox1.Text + "'" + "," + "'" + textBox2.Text + "'" + "," + "'" + textBox3.Text + "'" + "," + textBox4.Text+")"; OleDbCommand command = new OleDbCommand(query, connection); command.ExecuteNonQuery(); MessageBox.Show("Record Saved"); textBox1.Text = ""; textBox2.Text = ""; textBox3.Text = ""; textBox4.Clear(); textBox1.Focus(); } private void Form1_Load(object sender, EventArgs e) { //Code for database Connection string constring = "Provider=Microsoft.ACE.Oledb.12.0;Data Source=Mobile.accdb;"; connection = new OleDbConnection(constring); connection.Open(); } //CODE for Close button private void button1_Click(object sender, EventArgs e) { this.Close(); } private void Form1_FormClosing(object sender, FormClosingEventArgs e) { connection.Close(); } } }
Explanation of Phone Database Application
- The program is defined in the
MobileDB
namespace and contains a partial class namedForm1
, which represents the main form of the application. - The
Form1
constructor (public Form1()
) initializes the components of the form. - The
button2_Click
event handler is executed when the “INSERT” button (likely namedbutton2
by default) is clicked. It inserts a new record into the “Phone” table in the database.- It checks whether all text boxes (
textBox1
,textBox2
,textBox3
,textBox4
) contain values. If not, it displays a message. - It constructs an SQL query to insert data into the “Phone” table using the provided values from the text boxes.
- The
OleDbCommand
object is used to execute the query, andExecuteNonQuery()
is called to perform the insertion. - A message box informs the user that the record is saved.
- The text boxes are cleared, and focus is set to the first text box.
- It checks whether all text boxes (
- The
Form1_Load
event handler is executed when the form loads. It establishes a database connection using the OleDb connection string. - The
button1_Click
event handler is executed when the “Close” button (likely namedbutton1
by default) is clicked. It closes the application. - The
Form1_FormClosing
event handler is executed when the form is closing. It ensures that the database connection is properly closed before the application exits.
This program provides a basic interface to insert mobile phone records into a database table named “Phone.”