Temperature Conversion Visual Programming C Sharp – Design a Windows Forms Application in visual studio using C Sharp. Add a label for program header in a label control. Use a text box to input temperature to be converted. When we click on any one of the radio buttons with option To Celsius or To Fahrenheit, one option is selected. Click on Convert button to display converted temperature.
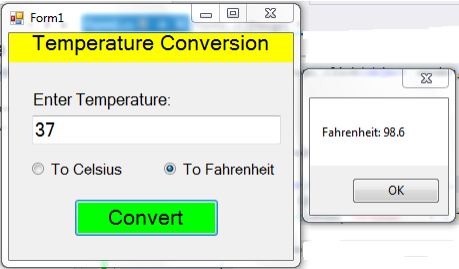
Source Code
using System; using System.Windows.Forms; namespace TemperatureConversion { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { double f, c; if (radioButton1.Checked == true) { f = double.Parse(textBox1.Text); c = (f - 32) * 5 / 9; MessageBox.Show("Celsius: " + c.ToString()); } else if (radioButton2.Checked == true) { c = double.Parse(textBox1.Text); f = (c * 9 / 5) + 32; MessageBox.Show("Fahrenheit: " + f.ToString()); } } }
Explanation of the Temperature Conversion Program in C Sharp
- The program is defined in the
TemperatureConversion
namespace and contains a partial class namedForm1
, which represents the main form of the application. - The
Form1
constructor (public Form1()
) initializes the components of the form. This constructor is responsible for setting up the GUI components and their initial properties. - The
button1_Click
method is an event handler that executes when the button labeled “button1” (likely named by default) is clicked. - Inside the
button1_Click
method:- The method starts by declaring two variables,
f
for Fahrenheit andc
for Celsius. - It checks which radio button is selected (Fahrenheit to Celsius or Celsius to Fahrenheit).
- If the “Fahrenheit to Celsius” radio button (
radioButton1
) is checked, the Fahrenheit value is parsed from the text intextBox1
, and the Celsius value is calculated and displayed using the conversion formula. The calculated Celsius value is shown in a message box. - If the “Celsius to Fahrenheit” radio button (
radioButton2
) is checked, the Celsius value is parsed fromtextBox1
, and the Fahrenheit value is calculated and displayed using the conversion formula. The calculated Fahrenheit value is shown in a message box.
- The method starts by declaring two variables,
- The conversion formulas for Fahrenheit to Celsius and Celsius to Fahrenheit are applied accordingly:
- Fahrenheit to Celsius:
(Fahrenheit - 32) * 5 / 9
- Celsius to Fahrenheit:
(Celsius * 9 / 5) + 32
- Fahrenheit to Celsius:
This program allows the user to input a temperature value in one unit (Fahrenheit or Celsius), choose the target unit for conversion, and then displays the converted temperature value in the other unit using a message box. It demonstrates a basic usage of Windows Forms and event handling in C#.
Python GUI Program Temperature Conversion Fahrenheit to Celsius
Program Temperature Conversion Algorithm Flowchart
C++ Program Temperature Conversion Celsius To Fahrenheit Formula