‘Python User Defined and Built-in Functions – Defining and Using Functions’ is our Python tutorial to explain the use of Python functions in Python programs / scripts.
We will cover the following topics on Python Functions:
Python Functions Defining and Using Functions
- What is a Function?
- Importance of Functions
- Advantages of Using Functions
- Types of Functions
- Builtin Functions
- User Defined Functions
- Defining a User Defined Function in Python
- General form of a Python Function
- Example of a Python Function to display Hello World
- Second Example of Function to display multipliation table
- Third Example of Function to Add Two Numbers
- Formal Parameters and Actual Parameters(arguments)
- Calling a Function with no returning value
- Three possible methods to call a function that returns a value.
- Python Code examples, The Complete Python Programs with output.
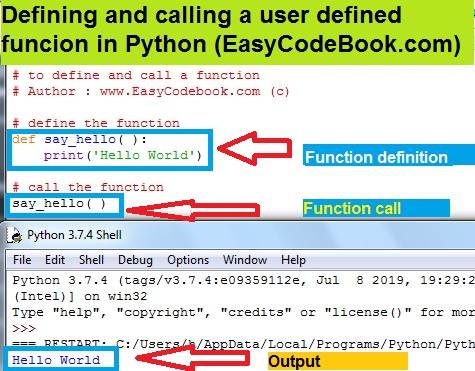
What is a Function?
A function is named block of code to perform some task. For example, in Python a print() function is a builtin function to display output on screen. Similarly, examples of some user defined functions are: a function to add two numbers, or a function to display factorial of a number.
Importance of Python Functions
Python functions support code resuability. If we need to write ‘a block of statements to perform some action’ in a program for many times. We will give a name to this block of statements. That is we will define a Python function by combining the name of function and the block of statements according to python rules.
Now whenever we need to repeat those statements in our Python code, we will just call that function by name.
Therefore, we can reuse the same code easily. It saves the prcious time of development. Moreover, using Functions can increase code readability and understandability.
Advantages of Using Python Functions
The advantages of using Python functions are as follows:
- We can divide a large program into small logical parts called functions. In this way we can write each smaller function more easily.
- We can modify each function easily and separately from other functions in a large program.
- Since functions are relatively smaller units of programs, so they can be easily maintained.
- Less programming time is needed to write a program with functions. Because different functions can be assigned to different programmers.
- When you have developed a function, it can be reused in your future code. Moreover you can share your functions with other developers like builtin functions of Python.
Types of Functions
There are two min types of functions
- Builtin Functions
- User Defined Functions
Builtin Function
Builtin functions are part of the Python language. They are provided to be used in our Python programs. For example, print() function in Python is used to display some output message to the user on the screen. For example, print(‘hello World’) will show a message Hello World on the screen.
Here you can find some important and useful Mathematical built-in functions with the example use in Python programs and the output.
User Defined Functions
We can define and use our own functions in Python easily. So these are called user defined functions. To use a function, we need to define it first.
The general Form of a User defined Function
def function_name(formal_parameter1,formal_parameter2,...): statement-1 statement-2 ... statement-n
Explanation of general Form of Python Function
First of all we start a function definition by keyword ‘def’. Furhter, we will write name of the function. We will provide a list of formal parameters separated by commas in paranthese. A colon is used after the right parantheses that indicate the beginning of block long with indentation. A block of indented statements will be provided on next lines. These statements must be indented. 4 spaces are recommended in the beginning of each line. When we wish to to end our function code, we will remove indentation from next statement.
Python User Defined and Built-in Functions
Example of a Simple Python function to display ‘Hello World’
This Function takes no argumnets and returns no value
def say_hello( ): print('Hello World')
How to Execute a Function: Calling a Function in Python
We will use a function call to execute a function. The gneral form of a function call of a simple function like say_hello( ) is
function_name()
Example:
say_hello( ) The output will be: Hello World
Formal Parameters and Actual Parmeters
Parameters are the values provided to the function for data processing. Formal Parameters are the variables used in function header. They receive data values from actual parameters. Actual parameters represent the actual values which are passed to a function. The function actually process these data items. Actual parameters are provided by the calling function in the function call. Actual parameters are also called arguments.
Calling a Python User Defined Function That Returns a Value
If a function returns a value, we will call such function, normally in the following three ways:
- Call a function in an Assignment statement
- In an output statement, print()
- And call a function in an expression
Calling a function In Assignment statement
The general form is:
variable_name = function_name(arguments)
The function will return a value that will be stored in the variable on left side of assignment statement.
Calling a Function in output statement like print()
The general form is:
print(‘Addition=’, add(100,200))
The function add will add 10 and 20 and will return 30 to be printed by print statement as:
Addition=300
Calling a function in Expression
One example is:
ans = add(10,20) + add(50,100)
Here the add function is called for two times. The first call will return 30, the second call will return 150. The both returned values will be added to give 180 that will be stored in ans valriable.
A Complete Python Program to show Calling methods of a function that Returns a value
# Python - User Defined Functions # Calling a function That Return a Value # Author: www.EasyCodeBook.com (c) # start of function definition def add(n1, n2): sum = n1+n2 return sum # the function definition ended with return statement # now we are calling # since the function will return a value # we call it in assignment statement to # store returning value in a vriabe ans print('Method 1: call the function in assignment statement') ans = add(10,20) # print the ans print('Addition=',ans) print('Method 2: call the function in print -output statement') print('Addition=',add(100,200)) print('Method 3: call the function in an expression') ans = add(10,20) + add(50,100) # print the ans print('Addition=',ans)
The output is: Method 1: call the function in assignment statement Addition= 30 Method 2: call the function in print -output statement Addition= 300 Method 3: call the function in an expression Addition= 180
What is a DocString or Document String
The first statement of the function body can optionally be a string literal. This string literal is called Doc String or document string of the function. The document string can be used to automatically produce online or printed documentation
Example of the document string:
"""Prints a Fibonacci series up to n."""
Second Example of Function to display multipliation table
This function takes a number and prints its table, returns no value
# An example Python program # to define and call a function table # to show multiplication table of a number # Author : www.EasyCodebook.com (c) # Note the first line in function is called # document string, it # define the function with formal # parameter num def print_table(num): """ This function prints multiplication table """ for i in range(1,11): print(num,' x ', i, ' = ',num*i) n=5 # call the function with actual # parameter n print_table(n)
The output of this program is:
5 x 1 = 5 5 x 2 = 10 5 x 3 = 15 5 x 4 = 20 5 x 5 = 25 5 x 6 = 30 5 x 7 = 35 5 x 8 = 40 5 x 9 = 45 5 x 10 = 50
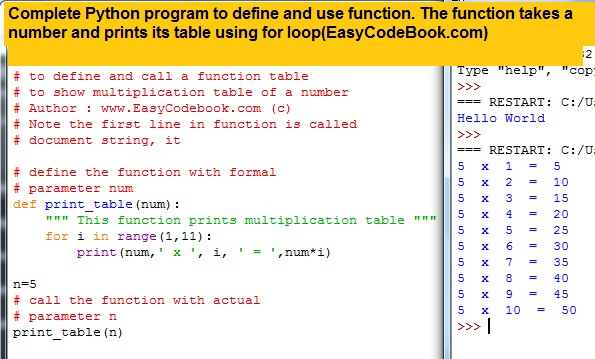
Display table Python function Complete Python program
3rd Example of a function add that Takes two arguments and Returns their sum
# An example Python program # to define and call a function add # takes two numbers, returns sum # Author : www.EasyCodebook.com (c) # Note the first line in function is a # document string. Docstrings are used to # automatically produce online or printed # documentation # define the function with formal # parameter num def add(num1, num2): """ This function takes two numbers returns sum """ return num1+num2 n1=5 n2=10 # call the function with actual # parameters n1,n2 result = add(n1,n2) print('Sum = ', result)
The out put will be:
Sum = 15
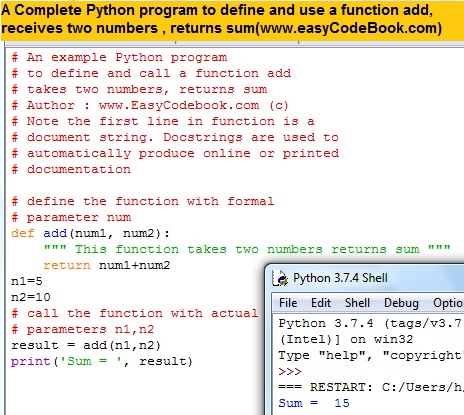
Python function add two numbers complete program
You will also like
- Python Recursive Function Check Palindrom String
- Python Insertion Sort Program using Array
- Python Array Module Selection Sort
- Python Bubble Sort using Array Module
- Python Multiplication Table of Number by Function Program
- C++ Inline Function Area of Circle
- Python GCD code using math.gcd() Function
- isprime Python Function Efficient Logic
- Python math Functions
- Python range Function in for loop
- Python print function – Printing Output
- Python input function to Get User Input
Hey there! This is my first visit to your
blog! We are a collection of volunteers and starting a new initiative in a community in the same niche.
Your blog provided us valuable information to work on. You have done a marvellous job!
Pingback: Python Local and Global Variables With Example Programs | EasyCodeBook.com
Howdy! This blog post could not be written much better! Going through this post reminds me of my previous roommate! He constantly kept talking about this. I most certainly will send this post to him. Fairly certain he’s going to have a great read. I appreciate you for sharing!
Pingback: Python Print Birthday Poem Program | EasyCodeBook.com
Pingback: Python Program Fibonacci Series Function | EasyCodeBook.com