Topic: Python Program Fibonacci Series Function
Write a user defined Fibonacci functin in Python to print the popular Fibonacci series up to the given number n. Here n is passed as an argument to the Fibonacci function and the program will display the Fibonacci series upto the provided number by the user input.
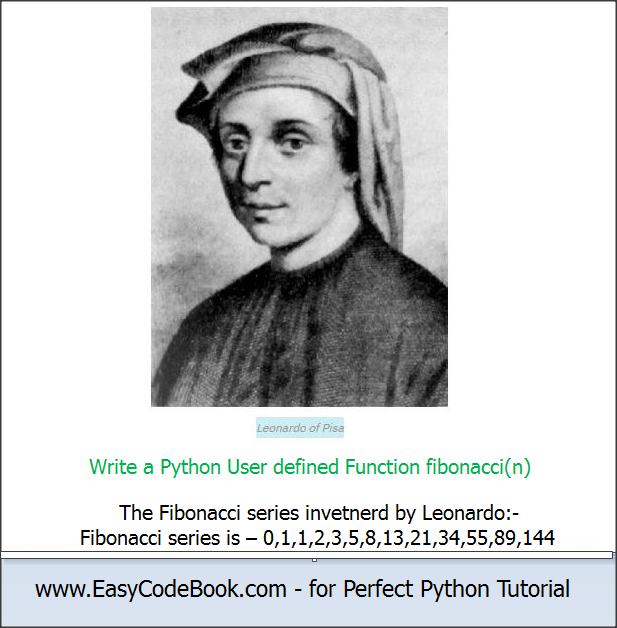
Python Fibonacci Series Function Program
What is a Fibonacci Series?
Definition of Fibonacci Series
The Fibonacci Sequence is the series of numbers, such that every next number in the fibonacci series is obtained by adding the two numbers before it:
Fibonacci series is – 0,1,1,2,3,5,8,13,21,34,55,89,144,233,377
Note: First two numbers in the Fibonacci series are 0 and 1 by default.
Examples:
Therefore third number or term 2 in the Fibonacci series is obtained by adding the two numbers before it that is, 0 and 1.
Similarly the fourth term 3 is obtained by adding the two numbers 1 and 2, before it, and so on.
Who invented the Fibonacci Series?
The popular Fibonacci series was invented by an Italian mathematician Leonardo of Pisa (aka Fibonacci) (1175 AD to 1250 AD.)
Why Leonardo Invented Fibonacci Series?
The real story behind the invention of the Fibonacci series is that: This series is invented by Leonardo because he was to solve a real life problem about the Rabbits farming. A rabbit farmer wanted to know how many rabbits he can grew in a year from one pair. The problem is solved by Fibonacci series by knowing and considering the following facts about the rabbit’s life. “A pair of rabbits bear another new pair in a single month. This new born pairs can bear another pair after the first month”.
Python Source Code for Python Fibonacci Series Function Program
# Write a Python 3 program to input a number n # and show the Fibonacci series up to that # number using a user defined function # www.EasyCodeBook.com # define the user defined function # to print the Fibonacci series up to n def fibonacci(n): """This function Prints the Fibonacci series up to n.""" a, b = 0, 1 while a < n: print(a, end=' ') a, b = b, a+b print() def main(): n = int(input("Enter a number n to show Fibonacci series upto n:")) fibonacci(n) main()
Output
Enter a number n to show Fibonacci series upto n:100 0 1 1 2 3 5 8 13 21 34 55 89 Enter a number n to show Fibonacci series upto n:1000 0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987
You would also like:
Python User Defined and Built-in Functions – Defining and Using Functions
C Program Fibonacci Series by Recursion
Fibonacci Sequence Generator Using Array C++
Fibonacci Program in Java to print n terms
Print Fibonacci Series Up to n – C Programming
Fibonacci Program in C Programming
- Python Recursive Function Check Palindrom String
- Python Insertion Sort Program using Array
- Python Array Module Selection Sort
- Python Bubble Sort using Array Module
- Python Multiplication Table of Number by Function Program
- C++ Inline Function Area of Circle
- Python GCD code using math.gcd() Function
- isprime Python Function Efficient Logic
- Python math Functions
- Python range Function in for loop
- Python print function – Printing Output
- Python input function to Get User Input