Task: Python Local and Global Variables With Example Programs – This Python programming tutorial explains the use of Python Local and Global Variables With Example Programs.
Using Local and Global Variables in Python Code
Local Variables in Python
What is a Local Variable in Python?
In Python any variable which is defined inside a function is called a local variable unless it is defiend expliciely global.
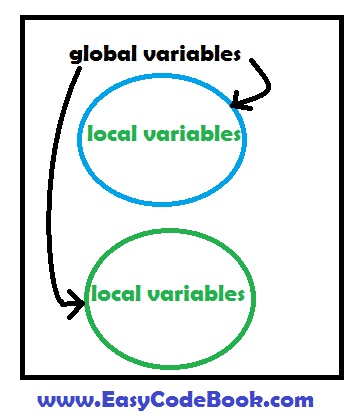
Python Local and Global Variables With Example Programs
For example, in the following Python code example, x is local but y is global variable.
def function1(): x=10 global y y=20
What is the Scope of a Local Variable?
The scope of a local variable is the body of the function in which it is dfined. A local variable cannot be accessed outside the function in which it is dfined.
For example, consider the following Python program. The first print() statement will access and display the value of local variable x. Since the print() statement and the local variable declaration is in the same function.
But the second print() will not be able to access x. Since the second print() is outside the body of function1. Therefore, it cannot access the variable x.
The error message is the result.
def function1(): x=10 print('x in function1 is =',x) # call the function function1() print('x=',x) # error Output - Error print('x=',x) # error NameError: name 'x' is not defined This error message is beacause we are trying to access x outside the function. But x is local variable to this function. Therefore we annot access x outside the function. If we try to do so we will get an error message NameError: name 'x' is not defined.
The Life Time of a Local variable
The life time of a local variable is equal to the execution time of the container function. As long as the function is running, its local variables exist. A local variable is created when it is defined in the function. This local variable will cease to exist when the function is terminated.
Whta is a Global variable in Python?
A global variable can be used in whole program, in all functions of a program.
How a Global Variable is defiend in Python
Python can define a global variable in either Implicitely or Explicitely.
When we define a variable outside any function, then Python makes it global implicitely. Now you can access this global variable in all functions of your program. Ths global variable is available to the whole program.
For example, the following Python code will give no error:
n=10 # n is global variable, since defined outside any function def function1() print('in function1, n=',n) def function2() print('in function2, n=',n) # call the functions function1() function2()
The output will be:
in function1 n= 10
in function2 n= 10
Explicit Declaration of Global variable in Python
Python uses a keyword ‘gloabal’ to declare global variables explicitely. The use of global keyword is compulsory when you are defining a global variable in a function. Otherwise the variable will be a local variable in the function.
Consider the following Python Code for example:
global n n=10 def function1() print('in function1, n=',n) def function2() print('in function2, n=',n) # call the functions function1() function2()
The output will be:
in function1 n= 10
in function2 n= 10
How to deine a Global Variable in a Function in Python
We use global keyword to define a global variable in a function. For example consider the following code:
def function1() gloabal n n=1000 print('in function1, n=',n) def function2() print('in function2, n=',n) # call the functions function1() function2()
The output will be:
in function1 n= 1000
in function2 n= 1000
What is the Scope of a Global Variable in Python
The scope of a global variable is the whole program. You can access a global variable in all functions and every where in the whole program.
Lifetime of a Global Variable
Lifetime of a global variable is equal to the execution time of the Python program in which we defined the global variable.
Python Local and Global Variables With Example Programs
global_var=100 def function1(): print('global_var in function1=',global_var) def function2(): print('global_var in function2=',global_var) # call function1 function1() # call function2 function2() # access global_var outside any user defined function print('global_var outside function=',global_var)
output global_var in function1= 100 global_var in function2= 100 global_var outside function= 100
# error def function1(): local_var1=10 print('Print local_var1 in function1=',local_var1) def function2(): print('Get local_var of function1 in function2=',local_var1) # call function1 function1() # call function2 function2() Output error Print local_var1 in function1= 10 Traceback (most recent call last): File "C:/Users/h/AppData/Local/Programs/Python/Python37-32/local1.py", line 16, in <module> function2() File "C:/Users/h/AppData/Local/Programs/Python/Python37-32/local1.py", line 9, in function2 print('Get local_var of function1 in function2=',local_var1) NameError: name 'local_var1' is not defined
Defining a global variable in a Function using global keyword # define global veriable explicitely def function1(): global global_var global_var=900 def function2(): print('Get global_var defined by keyword global of function1 in function2=',global_var) # call function1 function1() # call function2 function2()
output Get global_var defined by keyword global of function1 in function2= 900
Global outside function # define global veriable explicitely def function1(): global global_var global_var=900 global_var2=500 def function2(): print('Get global_var defined by keyword global of function1 in function2=',global_var) print(global_var2) # call function1 function1() # call function2 function2() output Get global_var defined by keyword global of function1 in function2= 900 500
Python Local and Global Variables With More Example Programs
You can access a global variable in function1, if global variable is defined after function1. # define global veriable explicitely def function1(): global global_var global_var=900 print('Implicite global variable defined outside function, global_var2=',global_var2) global_var2=500 def function2(): print('Get global_var defined by keyword global of function1 in function2=',global_var) print('Implicite global variable defined outside function, global_var2=',global_var2) # call function1 function1() # call function2 function2() output Implicite global variable defined outside function, global_var2= 500 Get global_var defined by keyword global of function1 in function2= 900 Implicite global variable defined outside function, global_var2= 500
You may also like to read a Perfect Python Tutorial on Using Functions in Python Programs