Python for loop Statement Syntax Plus Examples – This is a Python tutorial to explain the syntax and use of for loop with different practical examples in Python scripts.
Python for Statement
We can use a Python for statement for two main puposes:
- Use a for loop to iterate over items in a sequence
- Using for loop to use as a counter loop
Use a for loop to iterate over items in a sequence
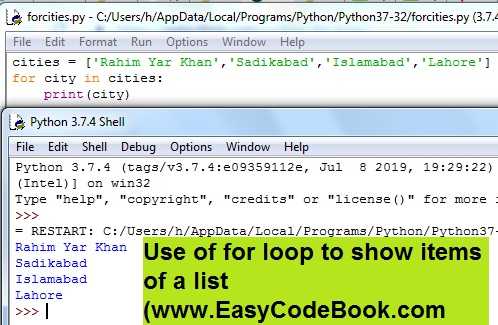
Python for statement syntax and Examples
Python for statement is generally used to iterate over a sequence of items like on a list or string. Therefore, we can display all characters in a string, or we can show all items in a list of cities.
When we use a for statemnt to iterate over a sequence of items, the general form of for statement is as follows:
Syntax of Python for Statement
for <item> in <sequence>: block_of_statements
Example of python for statement
Display names of all cities from a list using for statement:
Python Code is as follows:
cities = ['Rahim Yar Khan','Sadikabad','Islamabad','Lahore'] for city in cities: print(city) The output will be as follows:
Rahim Yar Khan Sadikabad Islamabad Lahore
Using a Python for statement as a Counter Loop
As we know that a counter controlled loop is used to repeat a set of statements for a fixed number of time. We may use a for statement to repeat a block of statements for a counter control variables’s initial value to final value. That is the block of statement is repeatedly executed as long as the given loop counter variable reaches its final value, like 10 times from 1 to 10.
Use of range() function in for statement
This is because of Python range() function. A range() function is used in for loop for this purpose, as follows:
Syntax of Python for Statement using range() function
for var in range(initial_value,excluding_final_value,step_value): block_of_statements
Note: Example: range(1,11,1), first 1 indicates initial value, 11 indicates excluding_final_value, means last value will be 10 not 11. 11 is excluding in the range. And last 1 indicates step value to increment. We can ignore 1 as it is default step value.
Example of for loop using range() function
Display 1 to 10 numbers using for loop in Python
for i in range(1,11): print(i)
The output will be:
1
2
3
4
5
6
7
8
9
10
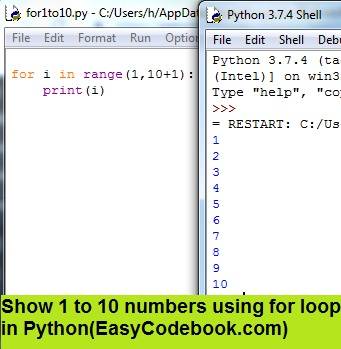
Python for loop syntax example to display 1 to 10
Why to Use 1 to 11 in range() function to get 1 to 10
We will use range(1,11) to display 1 to 10, because range function generates numbers from start to final excluding the final value. So if we use range(1,10), it will provide numbers from 1 to 9. Therefore to get numbers from 1 to 10, we use range(1,11) or range(1,10+1).
Another example of using Python for statement
Show all even numbers from 1 to 10 using Python for Statement
print('All even between 1 to 10 using for loop are:') for i in range(2,11,2): print(i)
Note: range(2,11,2) from 2 to 11-1 and increment / step of 2.
The ouput will be: 2 4 6 8 10
Example: Python for loop
Display 10 down to 1 numbers using for loop and range() function
print('Display 10 down to 1 using Python for loop,') for i in range(10,0,-1): print(i)
The output will be: Display 10 down to 1 using Python for loop, 10 9 8 7 6 5 4 3 2 1 Note: consider range(10,0,-1) once again. 10 is start, final value will be 1 (one) not zero as zero is excluded. And every time step will be of -1 that is decrement this time.
Another Syntax of for loop with else clause
Python for loop also contains an optional else clause. The block of statemnts related to else clause executed when for loop terminates normally completing its iteraions in a normal way.
Example: Show 1 to 5 using for loop else with clause
print('Display 1 to 5 using Python for loop with else clause,') for i in range(1,6): print(i) else: print('for loop terminated normally')
The output will be
Display 1 to 5 using Python for loop with else clause, 1 2 3 4 5 for loop terminated normally Note: that else cluase bloack of statements executed only onece after successful termination of loop.
If we use break Then else block not excuted
print('Display 1 to 5 using Python for loop with else clause,') for i in range(1,6): if i==3: break print(i) else: print('for loop terminated normally') print('for loop terminated with break statement')
Note: Since when i==3 , break statement will terminate for loop abnormally, so else clause block will not execute. And last statement which is not part of for loop will be executeed. it will display ‘for loop terminated with break statement’
The output of program:
Display 1 to 5 using Python for loop with else clause,
1
2
for loop terminated with break statement
Normally in a programming language we use for loop statemnt as a counter loop. The for loop iterates over a sequence of numbers, for example, to show 1 to 10 numbers in C++, we use the for loop as follows:
for(i=1; i<=10; i++) cout<<i<<endl;
But in Python a for statement is generally used for iterating over
items in a sequence like a list or string etc. For exaple accessing, traversing or displaying all characters in a string.
Consider an other example, we have a list of colors. We may use python for loop to print every item in this list.
Python for Loop Syntax Plus Examples
You will like to read about the use of continue and break statement in for loop as well as while loop.
Use of break and continue in Python with Examples
Python while loop Syntax Flowchart Example
Python range() Function in for loop
Pingback: Looping Statements in Python | EasyCodeBook.com
Pingback: Python Print Alphabet Patterns Programs | EasyCodeBook.com
Pingback: Use of break and continue in Python with Examples | EasyCodeBook.com
Pingback: Python pass Statement Example Code | EasyCodeBook.com
Pingback: Python Program to Print A to Z using for Loop | EasyCodeBook.com