Topic: Use of break and continue in Python with Examples
What is the importance of break and continue Statements in Python with Examples?
break Statement in Python
In Python programming language, break statement is used to break ( terminate ) the for loop or while loop. As soon as the break statement is executed the loop is ended and the conntrol will go to the statement immediately after the body of the loop.
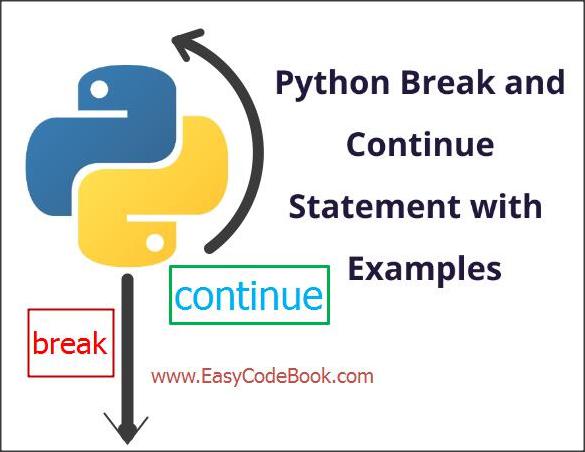
Use of break and continue in Python with Examples
Example of break statement
For example, we write a for loop as follows:
for num in range(1,11): print(num, end=' ') The output is: 1 2 3 4 5 6 7 8 9 10 Now we rewrite the above for loop but with break statement in an if statement: for num in range(1,11): if num==6: break; print(num, end=' ') Now the above oop will print 1 to 5 natural numbers, when num ==6 the condition becomes true and break statement is executed. The execution of the break statement within if statement will immediately terminate the for loop, therefore the output this time will be as follows: 1 2 3 4 5
A More Logical Example of break statement using while loop
We wish to enter and add some numbers repeatedly as long as we enter the numbers other than a ZERO. Therefore, input of 0 will terminate the while loop.
sum = 0 while True: num = int(input("Enter a number to add, [Input 0 to stop]: ")) if num == 0: break; sum += num print("Sum = ",sum) The output: RESTART: C:/Users/h/AppData/Local/Programs/Python/Python37-32/breakcontinue.py Enter a number to add, [Input 0 to stop]: 10 Enter a number to add, [Input 0 to stop]: 20 Enter a number to add, [Input 0 to stop]: 5 Enter a number to add, [Input 0 to stop]: 0 Sum = 35 How this output? The above while loop is an infinite loop, it will never terminate until we use a break statement. Therefore, when we enter a number other than zero, the statement sum += num will add it. If we enter a zero, the loop will terminate because of the execution of break statement.
continue statement in Python
On the other hand a continue statement in Python programming language will skip the remaining execution of the current iteration of the loop and the control will move to the next iteration of the loop.
Working of continue statement
We use the continue statement within the body of a loop. When the continue statement is executed, the remaining statements in the loop body are not executed for the current iteration. Now the control will go for the next iteration
Example of continue statement
Foe example, consider the following for loop:
for i in range(1,6): print(i) continue print('Hello Python World, www.EasyCodeBook.com') The output is: 1 2 3 4 5
How this Python code works?
This loop will execute the body of the loop for 5 times from i = 1 to 5. The first statement is print(i), it will print the value of i that is 1, 2, 3, 4, 5 on separate lines as shown above.
The second statement is continue, when executed, the control will skip the remaining statements of loop body:
print('Hello Python World, www.EasyCodeBook.com')
Hence the control will never execute this statement. Hence no output of Hello Python World, www.EasyCodeBook.com on the screen.
Another example using continue statement
for i in range(1,11) if i % 2 == 0: continue print i The output will be: 1 3 5 7 9 How this output? Since the loop body will be executed 10 times from i = 1 to 10. First of all the condition i%2 == 0 is as 1%2==0. Since 1%2 is equal to 1 so this condition is false, the cntinue will not execute. The control will go to the next statement ib loop body, which is a print(i), therefore, 1 is printed. Next time i = 2, hence the condition is true, 2%2 == 0. This time the continue will be executed and current iteration is skipped. Hence print(i) is not executed ( 2 is not printed on the screen). Similarly when the number is odd, 1, 3, 5, 7, and 9 the condition is false and continue is not executed. Therefore all odd numbers from 1 to 10 are shown. You will also like to read: Python pass Statement Example CodePython for Loop Syntax Plus Examples Python range Function in for loop Python while loop Syntax Flowchart Example
Pingback: Python for Loop Syntax Plus Examples | EasyCodeBook.com