Topic: Python 3 tkinter Message Widget Program Examples
What is a Message Widget
The Message widget is specially designed to show multiline non-editable messages. The message widget will adjust its width. It can wrap text too. It uses a single font to display a multiline message.
Why and When to use the Message Widget
We may use the message widget to display short text messages on multiple lines, using a single font.
Here is an example of a Python 3 GUI program to display a multiline colorful message on main window. It uses a message widget with some of its options like font, fore ground and back ground color, padx, pady, relief = GROOVE border decoration etc.
Python 3 tkinter Message Widget Program Examples
from tkinter import * def main(): main_window = Tk() main_window.title('Message') str_quote = "Real friends are hard to find, difficult to leave and impossible to forget." msg = Message(main_window, text = str_quote, padx=20, pady=20, bd=5, relief = GROOVE) msg.config(bg='green', fg='white', font=('verdana', 30)) msg.pack() mainloop() main()
How This GUI Program Works?
- Import * from tkinter GUI module.
- Define main function
- Create main window
- Set title of the main window
- Define a string quote
- Create a message object with different font properties
- Place message object in main window
Call the mainloop() to display the GUI and start the program. - Call the main function
You will also like:
- Python GUI Find Factorial by Recursive Function
- Python GUI Multiplication Table
- Python GUI Area of Triangle
- Python GUI Temperature Conversion Program C To F
- Python GUI Program Temperature Conversion Fahrenheit to Celsius
- Python Pounds to Kilogram Converter GUI tkinter Program
- Python 3 Four Function Calculator Program tkinter GUI
- Python Digital Clock Program using tkinter GUI
- Python Quotes Changer Program tkinter GUI
- Chnage Font Size Spinbox GUI Program Example
- Python 3 GUI Program tkinter Radio Buttons
- Adding Menus to Python 3 tkinter GUI Programs
Another simple GUI program example to show a message using Message Widget on multiple lines.
from tkinter import * def main(): master_window = Tk() str1="A Message for Python Programming Lovers" multiline_msg = Message(master_window,text=str1) multiline_msg.pack() mainloop() main()
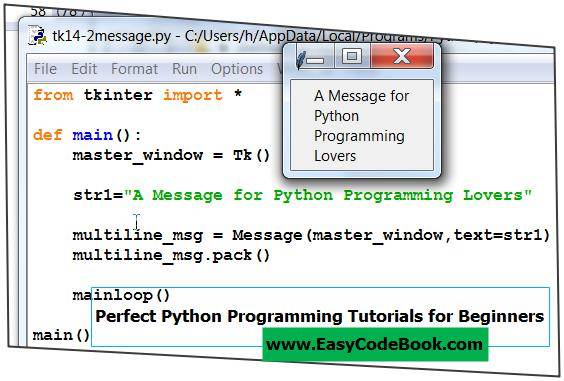
Python 3 tkinter GUI Message Widget Programs
Pingback: Find Factorial by Recursive Function Python GUI Program | EasyCodeBook.com
Pingback: Python 3 Radio Buttons GUI Program | EasyCodeBook.com