Input Validation in Python – Python Program to show number input validation.
Write a program that takes a number as input and handles any exceptions that may occur if the user enters a non-numeric value.
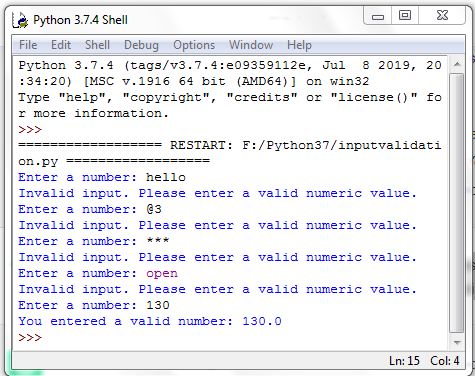
Source Code
while True: try: user_input = input("Enter a number: ") number = float(user_input) # If the input is successfully converted to a float, break out of the loop. break except ValueError: print("Invalid input. Please enter a valid numeric value.") print("You entered a valid number:", number)
Output
Enter a number: hello
Invalid input. Please enter a valid numeric value.
Enter a number: @3
Invalid input. Please enter a valid numeric value.
Enter a number: ***
Invalid input. Please enter a valid numeric value.
Enter a number: open
Invalid input. Please enter a valid numeric value.
Enter a number: 130
You entered a valid number: 130.0
In this program:
- We use a
while
loop to repeatedly prompt the user for input until they provide a valid numeric value. - Inside the
try
block, we attempt to convert the user’s input to a floating-point number usingfloat(user_input)
. - If the conversion is successful, the input is considered valid, and we break out of the loop.
- If the conversion raises a
ValueError
exception (i.e., the user entered a non-numeric value), we catch the exception in theexcept
block, display an error message, and continue the loop, prompting the user for input again.
Once the user enters a valid number, the program will display the number they entered.