Python Sentence Vowel Counter – Write a program that takes a sentence as input and counts the number of vowels (a, e, i, o, u) in it.
Here’s a Python program that takes a sentence as input and counts the number of vowels (a, e, i, o, u) in it:
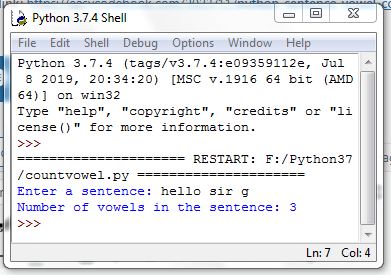
Python Sentence Vowel Counter Program
Source Code
# Function to count vowels in a sentence def count_vowels(sentence): vowels = "aeiouAEIOU" vowel_count = 0 for char in sentence: if char in vowels: vowel_count += 1 return vowel_count # Input from the user user_sentence = input("Enter a sentence: ") # Call the count_vowels function and display the result vowel_count = count_vowels(user_sentence) print("Number of vowels in the sentence:", vowel_count)
Output
Enter a sentence: hello sir g
Number of vowels in the sentence: 3
In this program:
- We define a function called
count_vowels
that takes a string (sentence
) as its argument. - Inside the function, we initialize
vowel_count
to 0. We also create a stringvowels
that contains all the vowels in both lowercase and uppercase. - We then iterate through each character in the
sentence
. For each character, we check if it exists in thevowels
string. If it does, we increment thevowel_count
by 1. - After processing the entire sentence, we return the
vowel_count
as the result. - The user is prompted to enter a sentence, and the
count_vowels
function is called with the user’s input. The program then prints the number of vowels in the sentence.
You can test this program with various sentences to count the number of vowels in each one.
- Python Count Each Vowel in Text File Program
- Python Remove Vowels in String Program
- Java Vowel Count in String Program
- Java Palindrome String Check
- Java String Class Reverse Program
- Check Vowel or Consonant Character C Programming
- Using Pointers Count Vowels