Python PyQt Gallons To Liters Conversion GUI Program – This code creates a simple PyQt GUI program with a main window, a label, an input field, a “Convert” button, and a label to display the result. When you enter the number of gallons and click the “Convert” button, it calculates the equivalent amount in liters and displays the result in the lower label.
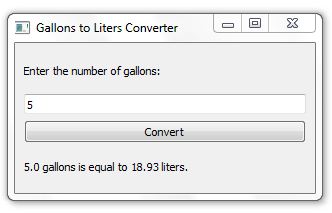
Python PyQt Gallons To Liters Conversion GUI Program
import sys from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QLabel, QLineEdit, QPushButton, QVBoxLayout class GallonsToLitersConverter(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle("Gallons to Liters Converter") self.setGeometry(100, 100, 300, 150) self.central_widget = QWidget() self.setCentralWidget(self.central_widget) layout = QVBoxLayout() self.label = QLabel("Enter the number of gallons:") self.textbox = QLineEdit() self.convert_button = QPushButton("Convert") self.result_label = QLabel() self.convert_button.clicked.connect(self.convert) layout.addWidget(self.label) layout.addWidget(self.textbox) layout.addWidget(self.convert_button) layout.addWidget(self.result_label) self.central_widget.setLayout(layout) def convert(self): try: gallons = float(self.textbox.text()) liters = gallons * 3.78541 self.result_label.setText(f"{gallons} gallons is equal to {liters:.2f} liters.") except ValueError: self.result_label.setText("Invalid input. Please enter a number.") def main(): app = QApplication(sys.argv) converter = GallonsToLitersConverter() converter.show() sys.exit(app.exec_()) if __name__ == '__main__': main()
To create a PyQt GUI program that converts gallons to liters, you’ll need to have PyQt installed. If you haven’t already installed PyQt, you can do so using pip:
pip install PyQt5
This PyQt-based program creates a Graphical User Interface (GUI) for converting gallons to liters. Let’s break down the program step by step:
- Import necessary modules:
sys
: Used for system-related operations.QApplication
,QMainWindow
,QWidget
,QLabel
,QLineEdit
, andQPushButton
are PyQt5 classes for creating the GUI elements.QVBoxLayout
is a layout manager used to arrange widgets vertically.
- Create a class
GallonsToLitersConverter
that inherits fromQMainWindow
. This class represents the main window of the application. - In the
__init__
method, initialize the application by calling theinitUI
method. - In the
initUI
method:- Set the window’s title and geometry (position and size).
- Create the central widget for the main window.
- Create layout manager
QVBoxLayout
to arrange the widgets vertically. - Create the following widgets:
label
: A QLabel widget displaying the text “Enter the number of gallons:”.textbox
: A QLineEdit widget for entering the number of gallons.convert_button
: A QPushButton widget labeled “Convert”.result_label
: A QLabel widget for displaying the conversion result.
- Connect the
clicked
signal of theconvert_button
to theconvert
method, which will be called when the button is clicked. - Add all the widgets to the layout and set it as the layout for the central widget.
- Create the
convert
method:- This method is called when the “Convert” button is clicked.
- It attempts to convert the user’s input to a floating-point number (
gallons
), then calculates the equivalent volume in liters, and displays the result in theresult_label
. - If the input is not a valid number, it displays an error message in the
result_label
.
- Define the
main
function:- Create a PyQt5 application (
QApplication
). - Create an instance of the
GallonsToLitersConverter
class, which represents the main window. - Show the main window.
- Start the PyQt event loop by calling
app.exec_()
, which allows the GUI to respond to user interactions.
- Create a PyQt5 application (
- Finally, the code checks if it’s the main module (not imported as a library), and if so, it calls the
main
function to start the application.
When you run this program, it will open a GUI window with a label, an input field, and a button. Users can enter a number of gallons, click the “Convert” button, and see the conversion result in liters displayed below the button. If the input is not a valid number, it will show an error message.