Python Program Print Star Diamond Shape –
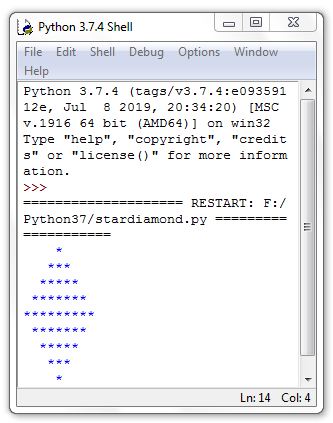
# Define the number of rows for the star shape num_rows = 5 # Loop to print the star shape for i in range(1, num_rows + 1): # Print spaces before the first half of the stars for j in range(num_rows - i): print(" ", end="") # Print the first half of the stars for j in range(2 * i - 1): print("*", end="") # Move to the next line print() # Loop to print the second half of the star shape for i in range(num_rows - 1, 0, -1): # Print spaces before the second half of the stars for j in range(num_rows - i): print(" ", end="") # Print the second half of the stars for j in range(2 * i - 1): print("*", end="") # Move to the next line print()
I’ll explain the code step by step:
num_rows = 5
: This line sets the variablenum_rows
to 5, which determines the number of rows in the star shape. You can change this value to create a star shape with a different number of rows.- The code uses two nested loops to print the star shape, one for the first half of the star and another for the second half.
- The first loop,
for i in range(1, num_rows + 1):
, iterates through the rows of the star shape. It starts from 1 and goes up to the specifiednum_rows
value. - Inside this loop, there’s a loop to print spaces before the first half of the stars. This loop,
for j in range(num_rows - i):
, calculates how many spaces to print before the stars in each row to create the pyramid shape. It prints spaces usingprint(" ", end="")
. - The next inner loop,
for j in range(2 * i - 1):
, prints the first half of the stars. It calculates the number of stars in each row based on the current row number and prints the stars usingprint("*", end="")
. - After printing the spaces and stars for the first half of the row, it moves to the next line using
print()
to start the next row. - After completing the first loop, there’s a second loop to print the second half of the star shape. This loop,
for i in range(num_rows - 1, 0, -1):
, iterates fromnum_rows - 1
down to 1, creating the inverted pyramid shape. - Inside this loop, there’s a loop to print spaces before the second half of the stars, similar to the first loop.
- The inner loop within this second loop prints the second half of the stars, again similar to the first loop.
- Finally, the code prints the spaces and stars for the second half of the row and moves to the next line.
The combination of these loops results in a star shape pattern with the specified number of rows, and the stars in each row are centered. You can adjust the num_rows
variable to change the size of the star shape.