Python Program Convert Gallons To Litres – To convert gallons to liters in Python, you can use the following simple program. The conversion factor is 1 US gallon = 3.78541 liters.
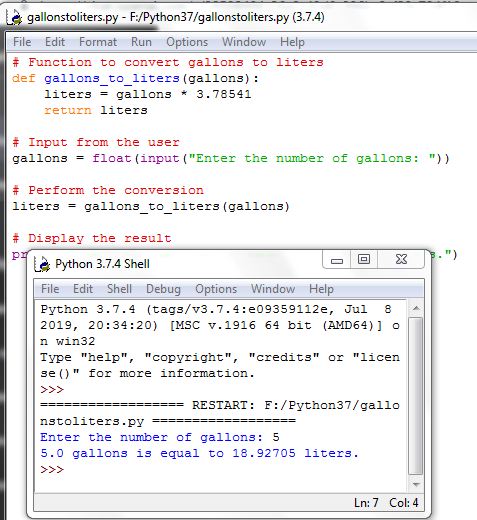
This program defines a function gallons_to_liters
that takes the number of gallons as input and returns the equivalent amount in liters. It then takes user input for the number of gallons, converts it to liters using the function, and displays the result.
# Function to convert gallons to liters def gallons_to_liters(gallons): liters = gallons * 3.78541 return liters # Input from the user gallons = float(input("Enter the number of gallons: ")) # Perform the conversion liters = gallons_to_liters(gallons) # Display the result print(f"{gallons} gallons is equal to {liters} liters.")
Let me explain the Python program in more detail:
- We start by defining a function
gallons_to_liters
that takes the number of gallons as its parameter and returns the equivalent volume in liters. It does this by multiplying the number of gallons by the conversion factor, which is 3.78541. This factor represents the number of liters in one US gallon. - We then prompt the user to input the number of gallons they want to convert to liters. The
input
function is used for this purpose. The input is accepted as a string, so we convert it to a floating-point number usingfloat()
so that we can perform mathematical operations with it. - Next, we call the
gallons_to_liters
function, passing the user’s input as an argument. The result is stored in the variableliters
. - Finally, we display the result to the user using the
print
function. The program prints a message that includes the original number of gallons entered by the user and the equivalent amount in liters, which is calculated using thegallons_to_liters
function.
For example, if the user enters 5 gallons, the program will calculate and print: “5.0 gallons is equal to 18.92705 liters.”
Here’s the formula to convert gallons to liters:
Liters = Gallons × 3.78541
Where:
- Liters is the equivalent volume in liters.
- Gallons is the volume in gallons you want to convert.
- 3.78541 is the conversion factor, representing the number of liters in one US gallon.
You can use this formula in your Python program to perform the conversion
This is how the program works to convert gallons to liters in Python.