Loop Invariant Concept and Uses
A loop invariant is a condition or property that holds true before and after each iteration of a loop in computer programming. It helps ensure that the logic of the loop is correct and that the desired outcome is achieved. Loop invariants are particularly important in proving the correctness of algorithms and programs.
Another Definition of Loop Invariant
A loop invariant is a statement about the variables in a loop that is true before and after each iteration of the loop. It is a property of the loop that is preserved throughout the execution of the loop.
Example of Loop Invariant
For example, consider the following code in Python to find the maximum value in an array:
def find_max(array): max_value = array[0] for i in range(1, len(array)): if array[i] > max_value: max_value = array[i] return max_value
The loop invariant for this code is that max_value
is always the maximum value in the subarray of array
that has been processed so far. This invariant is true before the loop starts, because max_value
is initialized to the first element of the array. It is also true after each iteration of the loop, because the loop only updates max_value
if it finds a larger value in the subarray.
Loop invariants can be used to prove the correctness of algorithms. For example, the correctness of the find_max()
function can be proved by showing that the loop invariant is true before and after each iteration of the loop, and that the loop terminates when the entire array has been processed.
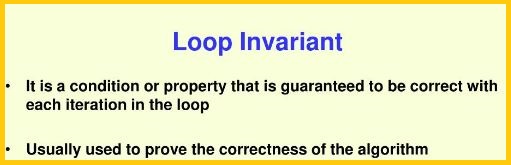
Loop Invariant Concept and Uses
How Loop Invariant Works
Here’s how loop invariants work:
1. Initialization
The loop invariant must hold true before the first iteration of the loop. This is typically established by initializing variables or data structures correctly before entering the loop.
2. Maintenance
During each iteration of the loop, the loop invariant should still hold true. In other words, if the invariant is true before an iteration, it should remain true after that iteration, assuming the loop body executed correctly.
3. Termination
When the loop exits, the loop invariant should still hold true. This ensures that the desired post-condition, which specifies what the loop should achieve, is satisfied.
Why We Use Loop Invariant?
Loop invariants are essential for understanding and reasoning about the behavior of loops and ensuring the correctness of algorithms.
Uses of Loop Invariant
They can be used for various purposes, including:
1. Debugging
Loop invariants can help identify issues in loop logic and quickly pinpoint where the code is going wrong.
2. Code correctness
By establishing and maintaining loop invariants, you can be confident that your loop will achieve its intended purpose.
3. Optimization
Loop invariants can sometimes provide insights into opportunities for optimizing loop performance by reducing redundant calculations or avoiding unnecessary work.
Example of Loop Invariant in C++ Programming Language
Here’s an example in C++ that demonstrates a loop invariant when calculating the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
int result = 1; // Initialize the loop invariant
for (int i = 1; i <= n; i++) {
result *= i; // Update result
// Loop invariant maintenance: result is still the factorial of i
}
// Loop invariant termination: result is the factorial of n
return result;
}
int main() {
int n;
cout << “Enter a non-negative integer: “;
cin >> n;
if (n < 0) {
cout << “Factorial is undefined for negative numbers.” << endl;
}
else {
int fact = factorial(n);
cout << “Factorial of ” << n << ” is ” << fact << endl;
}
return 0;
}
In this C++ example:
In this example, the loop invariant ensures that result
always contains the factorial of i
. This property holds true from the initialization phase to the maintenance phase and finally to the termination phase when the loop exits, ensuring the correctness of the factorial calculation.
The loop invariant is maintained throughout the loop, ensuring that the final value of `result` is indeed the factorial of `n`.
Second Example of a Loop Invariant – Sum of Array in C++
Here’s a simple example in C++ to illustrate the concept of a loop invariant. Consider a loop that calculates the sum of all elements in an array:
#include<iostream> using namespace std; int main() { int a[5] = {1, 2, 3, 4, 5}; // Initialize the loop invariant int total = 0; // Loop invariant: total is the sum of elements in a[0:i] for (int i = 0; i < 5; i++) { total += a[i]; // Update total // Loop invariant maintenance: total is still the sum of elements in a[0:i] } // Loop invariant termination: total is the sum of all elements in a cout << "The sum of all elements in the array is: " << total << endl; return 0; }
In this example, the loop invariant states that “total” is always the sum of the elements processed so far. It is true before the loop (initialization), during each iteration (maintenance), and after the loop (termination).
Loop invariants are a valuable tool in program correctness, and they play a crucial role in formal methods of program verification and algorithm analysis.