Constructor
In C++, constructors are special member functions that are automatically called when an object is created. They are used to initialize the object’s data members and perform any necessary setup tasks.
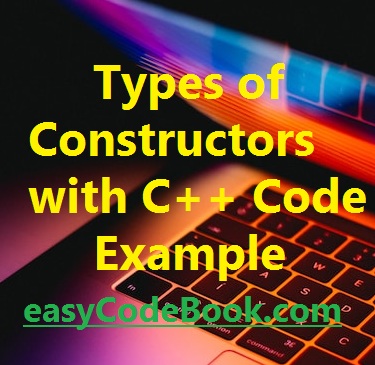
Types of Constructors in C++
There are different types of constructors in C++, each serving a specific purpose. Let’s explore four types of constructors with example programs:
1. Default Constructor
– A default constructor is a constructor that takes no parameters. If a class does not have any explicit constructors defined, the compiler generates a default constructor automatically.
– The default constructor initializes the object’s data members to their default values (e.g., 0 for numeric types, nullptr for pointers).
Example Program using Constructors in C++:
#include <iostream>
using namespace std;
class MyClass {
public:
int value;
// Default Constructor
MyClass() {
value = 0;
cout << “Default constructor called.” << endl;
}
};
int main() {
MyClass obj; // Default constructor is automatically called
cout << “Value: ” << obj.value <<endl;
return 0;
}
“`
2. Parameterized Constructor
– A parameterized constructor is a constructor that takes one or more parameters. It allows you to initialize the object’s data members with specific values provided during object creation.
Example Program:
#include <iostream>
using namespace std;
class MyClass {
public:
int value;
// Parameterized Constructor
MyClass(int val) {
value = val;
cout << “Parameterized constructor called.” << std::endl;
}
};
int main() {
MyClass obj(402); // Parameterized constructor is called with value 402
cout << “Value: ” << obj.value << endl;
return 0;
}
“`
3. Copy Constructor
– The copy constructor is a constructor that creates a new object by copying the values of another object of the same class. It is called when an object is passed by value or when an object is explicitly copied.
Example Program:
#include <iostream>
using namespace std;
class MyClass {
public:
int value;
// Parameterized Constructor
MyClass(int val) {
value = val;
}
// Copy Constructor
MyClass(const MyClass& other) {
value = other.value;
cout << “Copy constructor called.” << endl;
}
};
int main() {
MyClass obj1(10);
MyClass obj2 = obj1; // Copy constructor is called to create obj2 from obj1
cout << “Value of obj2: ” << obj2.value << endl;
return 0;
}
“`