Understanding Destructor in C++
What is a Destructor?
In C++, a destructor is a special member function of a class that is responsible for cleaning up and releasing any resources that the class might have acquired during its lifetime. It is automatically called when an object of the class goes out of scope, is deleted, or when the program terminates.
The destructor is a special member function that is automatically called when an object goes out of scope or is explicitly deleted using the `delete` keyword. It is used to perform cleanup tasks before the object is destroyed and its memory is deallocated.
Characteristics of Destructor in C++ Programming Language
Destructors have the following characteristics:
- Name and Syntax: A destructor has the same name as the class, preceded by a tilde (
~
). It has no return type and takes no parameters. The syntax for declaring a destructor is:~ClassName()
{
/* destructor code */
}
2. Automatic Invocation: Destructors are invoked automatically when the scope of an object ends or when it is explicitly deleted using the delete
keyword.
3. Resource Cleanup: Destructors are commonly used to release resources such as memory, file handles, database connections, or any other resources acquired by the object. This prevents memory leaks and ensures that resources are properly managed.
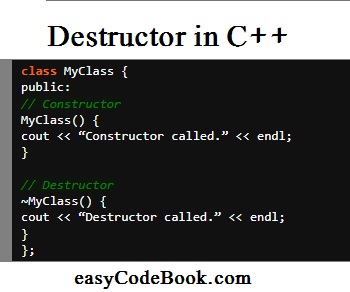
Understanding Destructor in C++
Example Program using Destructor
#include <iostream>
using namespace std;
class MyClass {
public:
// Constructor
MyClass() {
cout << “Constructor called.” << endl;
}
// Destructor
~MyClass() {
cout << “Destructor called.” << endl;
}
};
int main() {
MyClass obj; // Constructor is called
// The object will be destroyed when it goes out of scope
return 0; // Destructor is called before the program ends
}
Output:
Constructor called.
Destructor called.
——————————–
Process exited after 0.03532 seconds with return value 0
Press any key to continue . . .