This tutorial will explain the meaning and use of Const Functions Versus Non Const Functions with example C++ programs.
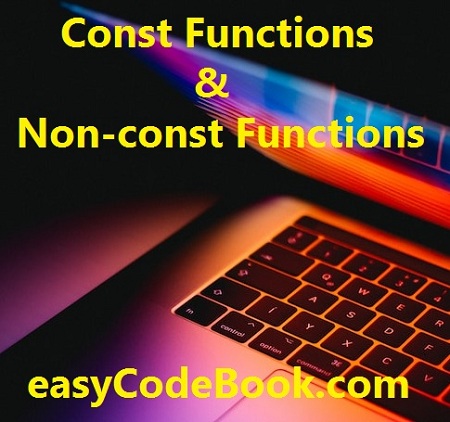
In object-oriented programming, “const” and “non-const” functions are two different types of member functions that operate on objects. These functions differ in their behavior and how they interact with the object and its data members.
1. Const Functions
- A const function is a member function that guarantees not to modify the state of the object it is called on. It is declared using the
const
keyword at the end of the function signature. - In other words, “Declaring a member function with the const keyword specifies that the function is a “read-only” function that doesn’t modify the object for which it’s called.
- Inside a const function, you cannot modify the values of non-static data members of the class (unless they are marked as
mutable
, which allows modification even in a const function). - Example:
The following class definition in C++ shows a const function named getValue(). It cannot change the state of the object. This means that it cannot modify data member 'value'. class MyClass { public: int getValue() const { // Cannot modify non-static data members here return value; } private: int value; };
2. Non-Const Functions
- A non-const function is a regular member function that can modify the state of the object it is called on. It does not have the
const
keyword in its signature. - Inside a non-const function, you can modify the values of non-static data members freely.
- Example:
- The following class definition in C++ shows a non const member function that can easily change the state of the object.
- It can modify the value of data member ‘value’.
class MyClass { public: void setValue(int newValue) { // Can modify non-static data members here value = newValue; } private: int value; }; Note: A const member function can be called by any type of object. Non-const functions can be called by non-const objects only.
Example C++ Program Using Const Member Function
#include<iostream>
using namespace std;
class MyClass {
int val;
public:
//constructor
MyClass(int x = 0) {
val = x;
}
// const member function
int getValue() const {
return val;
}
};
int main() {
const MyClass obj1(300);
MyClass obj2(800);
cout << "The value using object obj1 : " << obj1.getValue();
cout << "\nThe value using object obj2 : " << obj2.getValue();
return 0;
}
Output:
The value using object obj1 : 300
The value using object obj2 : 800
--------------------------------
Process exited after 0.06846 seconds with return value 0
Press any key to continue . . .
Example Program const and Non const Member Functions
#include<iostream>
using namespace std;
class MyClass {
int val;
public:
//constructor
MyClass(int x = 0) {
val = x;
}
// const member function
int getValue() const {
return val;
}
// non const member function
int setValue(int newValue) {
val = newValue;
}
};
int main() {
MyClass obj1(300);
obj1.setValue(1000);
cout << "The value using object obj1 : " << obj1.getValue();
return 0;
}
Output:
The value using object obj1 : 1000
--------------------------------
Process exited after 0.04821 seconds with return value 0
Press any key to continue . . .