Static Data Members and Functions in C++
In C++, static
is a keyword used to define class-level members that are shared among all instances (objects) of the class. These members are associated with the class itself rather than any specific object. There are two types of static members in C++:
Static Data Members
Definition: Static data members are class-level variables that have only one instance shared among all objects of the class.
- They are declared using the
static
keyword. - They are initialised outside the class definition.
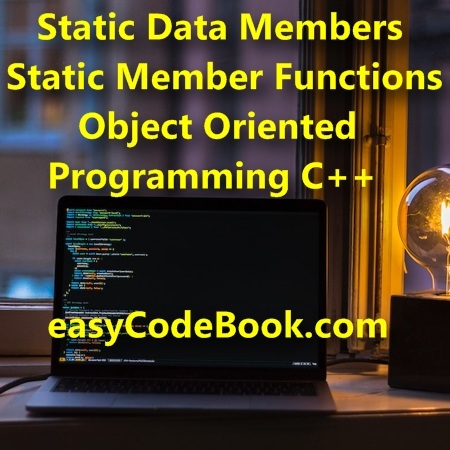
Static Data Members and Functions in C++
Why Static Data Members are Used?
- Static data members are usually used to store properties that are common to all objects of the class.
How Static Data Members are Accessed?
Accessing static data members is done using the class name instead of object instances.
Static Member Functions
definition: Static member functions are class-level functions that can be called without creating an object of the class.
-
- They are also declared using the
static
keyword and can only access static data members and other static member functions. - Since static member functions are not associated with any specific object, they don’t have access to non-static (instance) data members and cannot use
this
pointer.
- They are also declared using the
How To Access Static Member Functions?
Accessing static member functions is done using the class name, similar to accessing static data members.
Example C++ Program to Show the Use of Static Data Members and Static Member functions
Now let’s see an example of the Rectangle
class with a static data member and a static member function:
#include<iostream> using namespace std; class Rectangle { private: double length; double width; static int count; // Static data member to keep track of the number of Rectangle objects public: Rectangle(double len, double wid) : length(len), width(wid) { count++; // Increment the count whenever a new Rectangle object is created } static int getCount() { return count; // Static member function to return the count of Rectangle objects } }; // Initializing the static data member count int Rectangle::count = 0; int main() { Rectangle r1(5.0, 3.0); Rectangle r2(7.0, 4.0); // Accessing the static member function getCount() without creating objects cout << "Number of Rectangle objects: " << Rectangle::getCount() << endl; return 0; }
In this example, we added a static data member count to the Rectangle class, which is used to keep track of the number of Rectangle objects created. The count is initialized outside the class definition.
We also added a static member function getCount()
that returns the value of the static data member count
. This function can be called directly using the class name without creating any objects.
In the main
function, we created two Rectangle
objects, and using the Rectangle::getCount()
syntax, we accessed the getCount()
function to display the total number of Rectangle
objects created.
Output:
Number of Rectangle objects: 2
Static Data Members and Functions in C++