Function Overloading in C++
In C++, function overloading is a capability that enables the creation of multiple functions sharing the same name but having different parameter lists. When calling the function, the compiler determines the appropriate function to invoke based on the number or types of arguments passed.
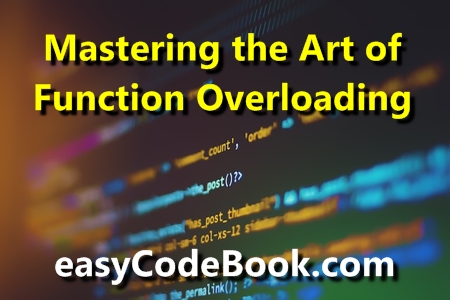
Mastering the Art of Function Overloading in C++
Example of Function Overloading in C++
Here’s an example program that demonstrates function overloading to add two integers, two doubles, and three integers:
#include<iostream> // Function to add two integers int add(int a, int b) { return a + b; } // Function to add two doubles double add(double a, double b) { return a + b; } // Function to add three integers int add(int a, int b, int c) { return a + b + c; } int main() { int intResult; double doubleResult; // Adding two integers intResult = add(5, 10); std::cout << "Addition of two integers: " << intResult << std::endl; // Adding two doubles doubleResult = add(3.14, 2.71); std::cout << "Addition of two doubles: " << doubleResult << std::endl; // Adding three integers intResult = add(1, 2, 3); std::cout << "Addition of three integers: " << intResult << std::endl; return 0; }
Output:
Addition of two integers: 15
Addition of two doubles: 5.85
Addition of three integers: 6
Explanation of Source Code
In this program, we have defined three overloaded functions named add
. The first add
function takes two integer parameters and returns their sum. The second add
function takes two double parameters and returns their sum. The third add
function takes three integer parameters and returns their sum.
During the program execution, the compiler will choose the appropriate add
function based on the arguments passed to it. The program will output the results of each addition operation.
Remember that the key to successful function overloading is having different parameter lists for the functions. The return type alone is not sufficient to distinguish between overloaded functions.
Static Polymorphism, Compile Time Polymorphism, Early Binding
Function overloading is a form of static polymorphism, compile-time polymorphism, or early binding. Static polymorphism refers to the ability of the compiler to determine which function to call at compile time based on the number or types of arguments provided to the function call.
In function overloading, multiple functions with the same name but different parameter lists are defined. The selection of the appropriate function to call is resolved by the compiler during the compilation phase based on the arguments used in the function call. That is why it is also called Static Polymorphism, Compile Time Polymorphism or Early Binding.
This is in contrast to dynamic polymorphism (runtime polymorphism or late binding), which is achieved using virtual functions and resolved at runtime using virtual tables and pointers.
Function overloading is an example of how C++ utilizes static polymorphism to provide different implementations for the same function name, allowing for more flexibility and readability in code while avoiding the complexity of virtual functions and runtime overhead.