Array Numbers Factorials in C++. This is a C++ program using arrays and loops.
This program will input five numbers in array. It will find factorials of each element of array and place them in a second array.
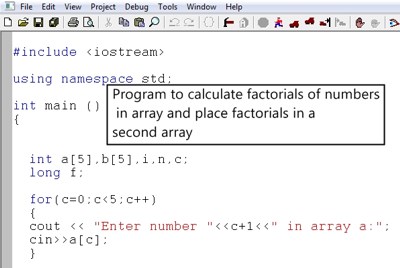
How This C++ Program Works?
1. First of all declare required arrays and variables.
int a[5],b[5],i,n,c; long f;
2. Input five numbers in array using a for loop from 0 to 4.
for(c=0;c<5;c++) { cout <<"Enter number "<<c+1<<" in array a:"; cin>>a; }
3. Use a for loop to access each number in the array. Calculate factorial of this number using another for loop. Place the factorial in second array.
// find factorial of each element of array for(c=0;c<5;c++) { n = a; f = 1; for(i=1;i<=n;i++) f = f * i; // place factorial in array b = f; }
4. display both arrays one after othe using separate for loops.
// display array cout<<"Array of numbers is : \n============\n"; for(c=0;c<5;c++) cout<<a<<"\t"; cout<<"\n"; // display array cout<<"Array of factorials is : \n===========\n"; for(c=0;c<5;c++) cout<<b<<"\t";
Source code of Factorials of Numbers in Array program in C++
#include <iostream> using namespace std; int main () { int a[5],b[5],i,n,c; long f; for(c=0;c<5;c++) { cout << "Enter number "<<c+1<<" in array a:"; cin>>a; } // find factorial of each element of array for(c=0;c<5;c++) { n = a; f = 1; for(i=1;i<=n;i++) f = f * i; // place factorial in array b = f; } // display array ara cout<<"Array of numbers is : \n============\n"; for(c=0;c<5;c++) cout<<a<<"\t"; cout<<"\n"; // display array cout<<"Array of factorials is : \n===========\n"; for(c=0;c<5;c++) cout<<b<<"\t"; return 0; }
Output from a sample run…
Enter number 1 in array a:1 Enter number 2 in array a:2 Enter number 3 in array a:3 Enter number 4 in array a:4 Enter number 5 in array a:5 Array of numbers is : ============ 1 2 3 4 5 Array of factorials is : =========== 1 2 6 24 120 Press Enter to return ...
More Programs …
Java Program Switch or Swap Arrays
Python Insertion Sort Program using Array
Python Array Find Average Temperature of Week Program
Python Array Find Minimum Number Program
Python Array Find Maximum Number Program
C Program Display and Count Odd Numbers in Array
C Program Find Sum of Even Numbers in Array
C Program Count Frequency of Each Element in Array
C Program Sum of Main Diagonal of Square Matrix
Insertion Sort Algorithm Trace Steps and C++ Program
Bubble Sort Algorithm Trace Steps on Sample Data and C++ Program