C Program Sum of Main Diagonal of Square Matrix
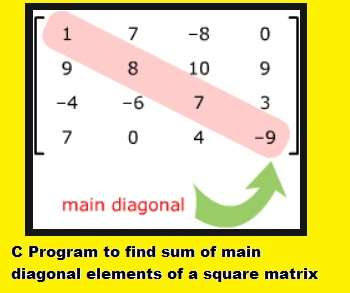
/* Write a C program to input a Square matrix of m rows and m columns and displaying the sum of the main diagonal elements from top left corner to right bottom corner. www.easycodebook.com */ #include <stdio.h> int main() { int sqmatrix[10][10]; int i,j,m,sum=0; printf("The number of rows and columns is same in a Square Matrix:\n"); printf("Enter number of Rows/Columns in Square Matrix[10 Maximum]:"); scanf("%d",&m); printf("\nEnter %d matrix elements in %d x %d Matrix:\n",m*m,m,m); for(i=0;i< m;i++) { for(j=0;j< m;j++) { printf("Enter element in Matrix[%d,%d]: ",i,j); scanf("%d",&sqmatrix[i][j]); } } printf("\nYou have entered the Square Matrix :\n"); for(i=0;i< m;i++) { for(j=0;j< m;j++) { printf("%d\t",sqmatrix[i][j]);/*print an element and a tab space*/ if(i==j) sum = sum + sqmatrix[i][j]; } printf("\n"); /*for new line after completion of one row*/ } printf("The Sum of the elements of Main diagonal\nof given Square Matrix is:%d",sum); return 0; }
Output The number of rows and columns is same in a Square Matrix: Enter number of Rows/Columns in Square Matrix[10 Maximum]:3 Enter 9 matrix elements in 3 x 3 Matrix: Enter element in Matrix[0,0]: 1 Enter element in Matrix[0,1]: 2 Enter element in Matrix[0,2]: 3 Enter element in Matrix[1,0]: 4 Enter element in Matrix[1,1]: 5 Enter element in Matrix[1,2]: 6 Enter element in Matrix[2,0]: 7 Enter element in Matrix[2,1]: 8 Enter element in Matrix[2,2]: 9 You have entered the Square Matrix : 1 2 3 4 5 6 7 8 9 The Sum of the elements of Main diagonal of given Square Matrix is:15
The Main Diagonal of a Square Matrix
The main diagonal of a matrix consists of those elements that lie on the diagonal that runs from top left to bottom right.
If the matrix is A, then its main diagonal are the elements who’s row number and column number are equal, that is i=j for two loop counter variables i and j for row and column respectively.
Given a 2D square matrix, find sum of elements in the main diagonals. For example, consider the following 3 X 3 input matrix. 1 2 3 4 5 6 7 8 9 Then the main diagonal consists of elements [0,0], [1,1], and [2,2]. Therefore, the sum of main diagonal elements is 1+5+9 = 15