Python while loop Syntax Flowchart Example – This Python programmingtutorial will explain in a simple and easy to understand way:
- The while loop statement in Python
- The Syntax of the while loop
- The flowchart of while loop
- The examples and working of while loop
- The Real Example of while loop
- The Python progam source code with output
Python while loop Syntax Flowchart Example
Definitin of while loop in Python
The while loop in Python execute a block of one or more statements as long the given condition remains true.
When to use a while loop?
A while loop is used when the user does not know in advance that for how many times the loop body will execute.
Therefore, a while loop is important when we do not know the exact number of iterations in advance.
The Syntax of while loop in Python
The general form of a Python while loop is as follows:
while condition: indented-statemnt-1 indented-statemnt-2 ... last-indented-statement
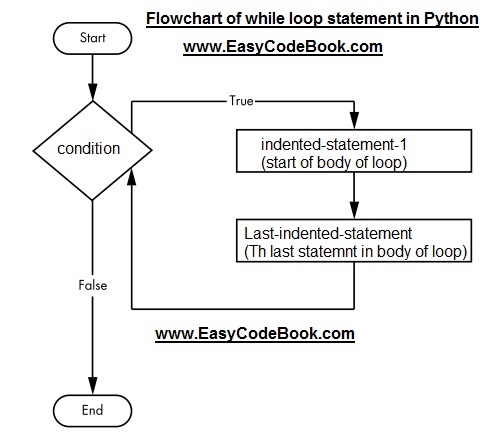
Python while loop statement, syntax, flowchart, working, real world examples
Explanation of the Syntax of while loop
- while is a reserved word.
- condition is a boolean expression that gives a True or False result
- a colon :
- A block of one or more indented statements represents the body of the while loop. It means that, the set of statements that will be executed as long as the given condition remains true. We also call the block of statements a ‘while clause’.
- Note: indented statements are included in loop body, and if a statement is written with no indentation after last-indented-statement, this will not be included in the body of loop.
- Intialization and Increment / decrement of loop control variable
We must remember that we will intialize the loop control variable before the while loop statement. Moreover, we must take care to change the value of a loop control variable inside the blck of statements (loop body).
The change of value means either incrementing the value or decrementing the value in some way, so as the while loop will terminate at last. If we do not perform some action in loop body, there is a chance that the loop will becomes an infinite loop.
Working of the while loop statement in Python
First of all, the given condition is tested, if it is true, the control will excute the while clause that is body of loop.
After executing the whole block, the condition is tested again. If it is true, then the control will execute the body of loop once again. This process continues as long as the given condition remains true. The loop is terminated if the given condition becomes false.
A Simple Example of while loop statement in Python
First, we will use a simple example to undrstand the syntax and working of the while loop.
Display 1 to 5 numbers using a while loop.
i = 1 while i<=5: print(i) i+=1 print('This is a next statement to while loop')
The output will be:
1 2 3 4 5 This is a next statement to while loop
How this while loop Works?
1. First of all, we initialize the loop control variable i to 1, before starting the while loop.
2. Now the condition is tested, since the given condition is true, 1<=5, the control will execute the loop body.
Rememebr, the loop body consists of two indented statements: print(i) and i+=1.
3. print(i) prints the value of i that is a 1 on the screen and advances the output control to next line.
4. i+=1 will increment the value of i by 1, so that i is equal to 2.
5. The next statement in not indented, it means that the while clause or loop body is ended after the statement i+=1 statement.
This was the first iteration of the while loop. Now the second iteration will start in the same way.
The condition is true, 2<=5, therefore the loop body will execute again. The prnt(i) will print 2. The i+=1 increases the vale of i, sothat i=3 now.
The Third Iteration of while loop
3<=5 is true, so execute loop body, print(i) prints 3. i+=1 sets i=4.
The 4th Iteration of the while loop
4<=5 is true, so execute the loop body, print(i) prints 4, i+=1 sets i=5
The 5th iterationof the while loop
5<=5 is tru, so execute the loop body. print(i) will print 5, i+=1 sets i=6
The condition will be tested again. 6<=5 is false now. Hence the control will not execute the loop body. This terminates the while loop and the control will move to the next sttement immidiately after the while loop.
Example 2:
Display Hello World five times using the while loop. Draw a flow chart for this while loop also.
Solution:
i = 1 while i<=5: print('Hello World') i+=1
The output will be: Hello World Hello World Hello World Hello World Hello World
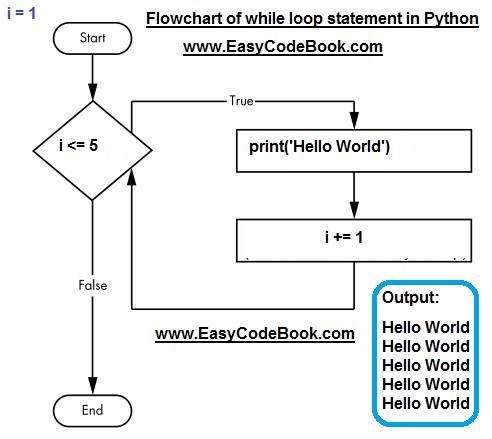
Python while loop statement examples
The Real Example of a while loop statement
We know that the while loop is especially useful when we do not know the exact number of iterations in advance. Therefore, we must explain this with a real example that fulfils this condtion.
The problem statement: A user have to find the sum of many numbers. Write a while loop to display a message ‘Eneter a number or -1 to stop’. The user will enter a number as long as he wishes to continue to add numbers. Finally, he will enter -1 to stop the process and find the sum.
Solution:
# Write a Python program for the following: # The problem statement: A user have to find the total sum # of many numbers. Write a while loop to display a # message 'Eneter a number or -1 to stop'. # The user will enter a number as long as he wishes # to continue to add numbers. Finally, he will enter -1 # to stop the process and find the sum. # # Author : www.EasyCodeBook.com num=0 total=0 while num!=-1: num = int(input('Enter a number to add or enter -1 to stop:')) if num!=-1: total = total + num print('The sum of all numbers is:',total)
The output will be: Enter a number to add or enter -1 to stop:5 Enter a number to add or enter -1 to stop:10 Enter a number to add or enter -1 to stop:5 Enter a number to add or enter -1 to stop:1 Enter a number to add or enter -1 to stop:4 Enter a number to add or enter -1 to stop:-1 The sum of all numbers is: 25
Would you like to dare to solve the following problem with while loop
Write a Python program /script so that the user can enter as many numbers as he wishes for finding the sum and average. The loop will be terminated when he will enter a -1. Finally the program will calculate and show the total and average of numbers.
The else clause in while loop
An else clause is an optional clause in the while loop. It is executed only once when the given condition of loop becomes false and the loop is terminated normally.
The loop body is executed as long as the given condition remains true. Whereas, the else clause will be executed if the condition becomes flase.
The Syntax and Example of while loop with else clause
while condition: indented-statemnt-1 indented-statemnt-2 ... last-indented-statement else: else-statement-1 .... last-else-statement
i = 1 while i<=5: print('Hello World') i+=1 else: print('The while loop terminated normally')
This code will produce the output: Hello World Hello World Hello World Hello World Hello World The while loop terminated normally
i = 1 while i<=5: if i==3: break; print('Hello World') i+=1 else: print('The while loop terminated normally')
This code will show Hello World two times only. When i is 3 the loop will be terminated due to break statement. Hence else clause will not be executed now. Hello World Hello World
whoah this blog is fantastic i really like studying your articles. Stay up the good work! You know, many individuals are hunting around for this information, you can aid them greatly.
I need to to thank you for this great read!! I definitely enjoyed every
bit of it. I’ve got you book-marked to look at new stuff you post…
Pingback: Python for Loop Syntax Plus Examples | EasyCodeBook.com
Pingback: Use of break and continue in Python with Examples | EasyCodeBook.com