“C File Program to Save Records” is a file handling program in C programming language. This program will open a binary file and save the records of students in it. If the file does not exist already, it is created.
It will add one record at a time. After saving first record successfully in a file, it will ask from user to ‘Do you want to add more records – Press y or n=’. If the user enters ‘n’ then program will be terminated. Otherwise the program will ask the user to enter a new record of student.
Similarly after every record entered, it will ask the user again.
We will use the following C statements to accomplish the given task of storing the records of students in a C File in binary format.
Declare a File Pointer
We will use the statement
FILE *fptr;
to declare a file pointer.
Open File
We use a library function fopen() to open a file in a specific mode for reading, writing or appending etc.
fptr = fopen(“filename”,”open-mode”);
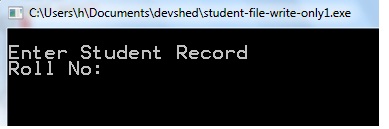
Ask to user for entering information for Record
If file is opened successfully, the program will ask the user to enter necessary information to record.
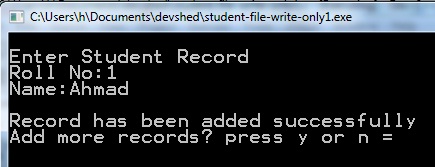
C File program to write records in binary file
Writing a single Record to Binary File
After getting the information from the user, the program will use another library function known as fwrite() to write / store the record in the binary file.
fwrite(&srecord, sizeof(srecord), 1, fptr);
Wish to add more Records? Press y / n ?
After writing the given record into binary file, the program will ask the user to press ‘y’ if he wishes to add more records or press ‘n’ to stop.
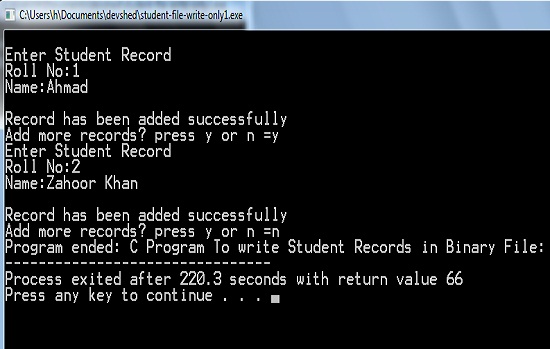
how to Write records in binary fie c filing
Closing a File
We use a standard library function known as fclose() to close the opened binary file
fclose(fptr);
In this way our C program to save records in a binary file, reaches to end.
The source code of the C File program for writing / storing records in a binary file:
#include<stdio.h> #include<conio.h> #include<stdlib.h> struct student { int rollno; char name[30]; }srecord; int main() { FILE *fp; char ans; int i; /*opening binary file in appending / writing mode*/ fp=fopen("d://sfile.dat","ab"); if(fp==NULL) { printf("File could not open"); exit(0); } while(1) { printf("\nEnter Student Record\n"); printf("Roll No:"); scanf("%d",&srecord.rollno); getchar(); printf("Name:"); gets(srecord.name); /* write a record to binary file */ fwrite(&srecord,sizeof(srecord),1,fp); printf("\nRecord has been added successfully"); printf("\nAdd more records? press y or n ="); ans=getche(); if(ans=='n' || ans=='N') break; } fclose(fp); printf("\nProgram ended: C Program To write Student Records in Binary File:"); return 0; }
Pingback: C Program to Read Records From Binary File » EasyCodeBook.com
Pingback: C Program to Append / Add More Records in Binary File » EasyCodeBook.com
Pingback: Display Yearly Calendar C Program | EasyCodeBook.com