Here is a C Program to Print Fibonacci Series Up to n. Where n is the given number provided by the user at run time. Therefore, if the user inputs a number 10, the output of this Fibonacci series program will be:
0, 1, 1, 2, 3, 5, 8
Note that the last term of Fibonacci series is 8 which is less than the given number 10.
Here is an image to show the sample run and output of the C Program To print Fibonacci series up to n:
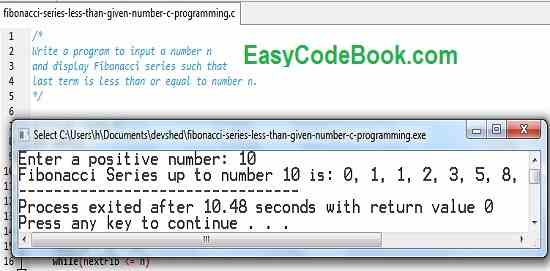
The source code for this C program to print Fibonacci numbers up to the given number n is as follows:
/* Write a program to input a number n and display Fibonacci series such that last term is less than or equal to number n. */ #include <stdio.h> int main() { int n, t1 = 0, t2 = 1, nextFib; printf("Enter a positive number: "); scanf("%d", &n); // print the first two terms by definition, 0 and 1 printf("Fibonacci Series up to number %d is: %d, %d, ",n, t1, t2); nextFib = t1 + t2; while(nextFib <= n) { printf("%d, ",nextFib); t1 = t2; t2 = nextFib; nextFib = t1 + t2; } return 0; } /* Output of Fibonacci Series upto given number program in C language is: Enter a positive integer: 10 Fibonacci Series up to number 10 is: 0, 1, 1, 2, 3, 5, 8, */
We may see an example output in the source code of the program. Similarly, the output of this program is also present in an image used in this post.
After typing this program in Dev C++ IDE for C and C++, the user will compile the program. If there is no error, the compiler will generate object code. The linker will convert the object code into executable code after linking necessary library files. In the end the user will execute the program and the output screen will appear.
You may also like a related program:
Write a C program to show Fibonacci series up to given number of terms entered by user.
You may also like Fibonacci Series up to n terms Program in C++