C Program to Display Records From Binary File
“Read Records From Binary File” in C Programming is the program we will write today. We have already written a C program to Write Records in a Binary File. We have learned some basic standard library function used in C File programming.
- fopen() to open a file.
- fclose() to close a file
- fread() to read records one by one from a binary file. etc.
Syntax and use of fread() library function for files
Similarly, a standard library function in C programming language is known as fread() function. fread() has parameters similar to fwrite function. For example
fread ( &srecord, sizeof(srecord), 1, fp);
fread() function reads one record at a time, so we will put this function in a while loop. This while loop will continue to read one record at a time as long as fread() function is able to read a record. Therefore, This file reading while loop will break when end of file will be reached.
How many Records to Read and Display?
Since we have entered only two records in C File Program to write records in binary file. Therefore, these two records will be displayed on screen.
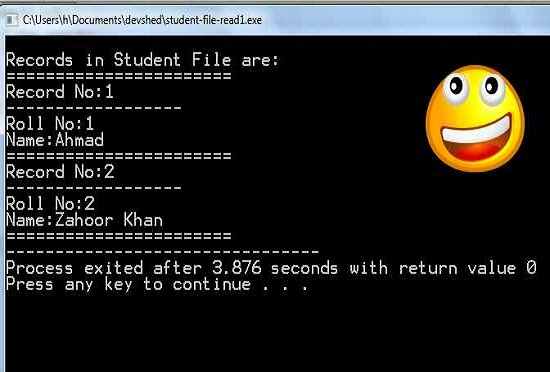
We may view the output screen of C program to write records in binary file. This confirms that we have inserted only two records in student binary file. We added these records when we executed a File Program to write records in a binary file. We used fwrite() function to write records in a binary file.
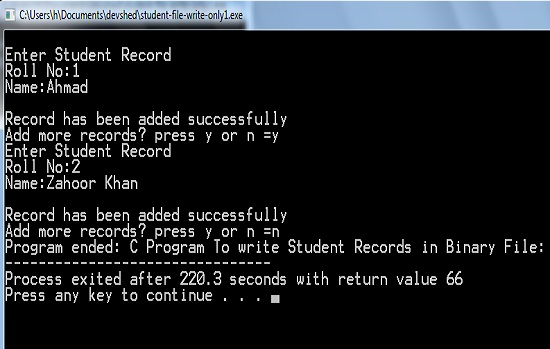
output screen of C program to write records in binary file.
Source code of c program to read and show all records from a binary file.
/* Write a C program to read a binary file and show all records on screen */ #include<stdio.h> #include<conio.h> #include<stdlib.h> struct student { int rollno; char name[30]; }srecord; int main() { FILE *fptr; char ans; int i; /*open binary file in read mode*/ fptr=fopen("d://sfile.dat","rb"); if(fptr==NULL) { printf("File could not open"); exit(0); } printf("\nRecords in Student File are:"); printf("\n======================="); i=1; while((fread(&srecord,sizeof(srecord),1,fptr)==1)) { printf("\nRecord No:%d",i); printf("\n------------------"); printf("\nRoll No:%d",srecord.rollno); printf("\nName:%s",srecord.name); i++; printf("\n======================="); } fclose(fptr); }
Pingback: C Program to Append / Add More Records in Binary File » EasyCodeBook.com