Python GCD Program with for statement – This Programming tutorial will explain a simple logic and Python code to find GCD. A simple for loop statement is used to calculate Greatest common Divisor of the two given numbers.
The Source code and Output of Python GCD Program with for statement
# Write a Python program to find # GCD of two numbers # # Author: www.EasyCodeBook.com (c) num1= int(input('Enter the first number=')) num2= int(input('Enter the second number=')) if num1<num2: smaller = num1 else: smaller = num2 for i in range(1,smaller+1): if num1 % i == 0 and num2 % i == 0: gcd = i print('GCD of',num1,'and',num2,'is:',gcd)
Output of Python GCD Program
The output is: Enter the first number=20 Enter the second number=30 GCD of 20 and 30 is: 10 Another sample run: Enter the first number=45 Enter the second number=54 GCD of 45 and 54 is: 9
Define GCD with Examples
GCD is a short for Greatest Common Divisor. Therefore, GCD of two numbers is the largest number that divides both the given numbers.
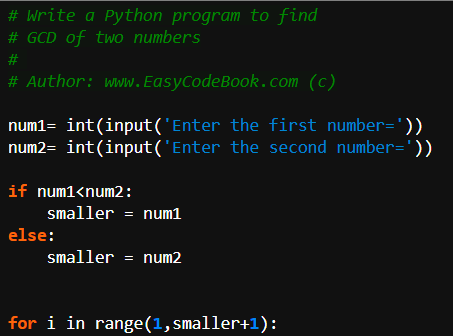
Example of GCD of Two numbers Calculation
Problem: Find the GCD of 20 and 30.
Step 1: Find the divisiors of given numbers:
The divisiors of 20 are : 1, 2, 4, 5, 10,20
The divisiors of 30 are: 1, 2, 3, 5, 6, 10,15,30
Step 2: Find the greatest number which is common in both lists, hence GCD is 10.
The Programming Logic to Find GCD of Two Numbers with for statement
1. First of all we find the smaller of the two numbers. For example, 20 is smaller than 30.
2. Now we start a loop from 1 to the smaller number. For example from 1 to 20.
3. We will divide the given numbers num1 and num2 by i.
4. Whenever i will divide the both numbers evenly, we set gcd=i
5. Therefore at the end gcd=10 which is the last and greatest common divisor of both 20 and 30.