Looping Statements in Python – This tutorial explains the two types of loops in Python briefly.
What are looping Statements in Python?
Looping statements are used to execute a block of one or more statements repeatedly for a fixed number of times or as long as a given condition remains true.
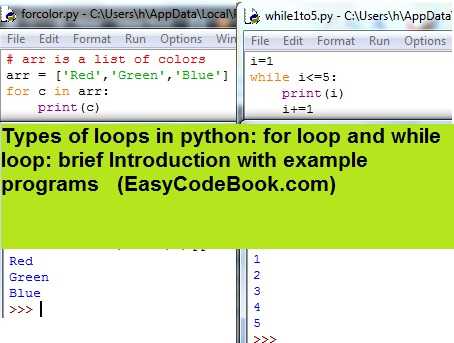
types of python looping Statements- for and while loop
Types of Python Looping Structures
There are two main loop statements in Python as follows:
- while loop
- for loop
Python while Statement
Python provides a while loop statement. A while loop is used to repeatedly execute a block of one or more statements as long as a given condition remains true. The while loop terminates, if the given condition becoms false.
Example:
i=1 while i<=5: print(i) i+=1
The output will be: 1 2 3 4 5
The Python for Statement
The Python for Statement is used to iterate over the members of a sequence in order, executing the block of statements each time.
For example:
# arr is a list of colors arr = ['Red','Green','Blue'] for c in arr: print(c)
The output will be:
Red
Green
Blue
Looping Statements in Detail
Click the following links for detailed tutorials on for loop and while loop:
Python for Loop with Syntax and Examples in Detail
Python while statement with Syntax and Examples in detail
Pingback: Python Sentinel Controlled Loop Example Program | EasyCodeBook.com
Pingback: Python Print Alphabet Patterns 11 Programs | EasyCodeBook.com
Pingback: Python pass Statement Example Code | EasyCodeBook.com