Task: Java switch Statement Month Days Program – This Java Program will input a Month Number from the user at run time. Then this Java program will display the number of days for th input month number. If the month number is not between 1 and 12, then this java program will display an error message ‘Invalid Month Number.
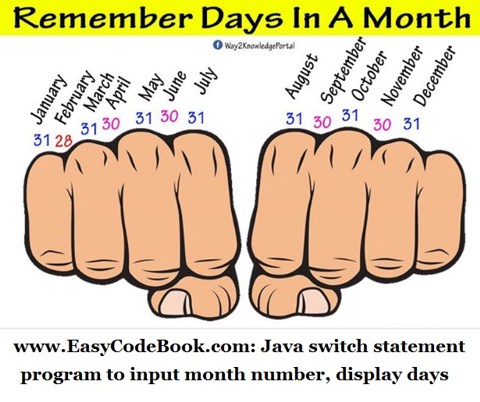
Java switch Statement Month Days Program
The Source Code of Java Month Days Program
/* * Write a Java Program using switch statement. * To input a Month Number from user using Scanner classs. * This Java program then displays the number of days in this month. * If number is not between 1 and 12 then program will display * Invalid Month Number message */ package monthdays; import java.util.Scanner; /** * * @author www.EasyCodebook.com */ public class MonthDays { /** * @param args the command line arguments */ public static void main(String[] args) { int n; Scanner input=new Scanner(System.in); System.out.print("Enter month number:"); n=input.nextInt(); switch(n) { case 1: System.out.println("January has 31 days"); break; case 2: System.out.println("February has 28 days"); break; case 3: System.out.println("March has 31 days"); break; case 4: System.out.println("April has 30 days"); break; case 5: System.out.println("May has 31 days"); break; case 6: System.out.println("June has 30 days"); break; case 7: System.out.println("July has 31 days"); break; case 8: System.out.println("August has 31 days"); break; case 9: System.out.println("September has 30 days"); break; case 10: System.out.println("Octuber has 31 days"); break; case 12: System.out.println("December has 31 days"); break; default: System.out.println("Invilid number"); } } }
The output of Java switch Statement Program
Enter month number:6
June has 30 days
Enter month number:15
Invilid Month Number
Enter month number:3
March has 31 days