Task: Write a Java program to create a new Box class in Java. Use three instance variables width, height and depth of type double. Write down two constructors. Default ( no-arg )constructor will intialize the Box class objects with 0.0 value for all instance variables. A parameterized constructor will be used to intialize the object with different values.
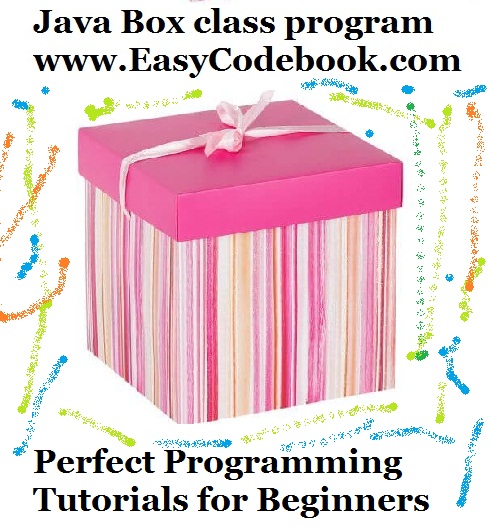
Write down a method called volume() that will calculate and show the volume of the box.
Write down a main class to use this Box class. Create some objects of Box class and show the functionality.
Java Source code for designing and using a new class Box
/* Write a Java program to create a new Box class in Java. */ package boxdemo; /** * * @author www.EasyCodeBook.com */ class Box { private double width; private double height; private double depth; public Box() { width=height=depth=0.0; } public Box(double w,double h,double d) { width=w; height=h; depth=d; } public void volume() { System.out.println("Volume ="+(width*height*depth)); } } public class BoxDemo { public static void main(String[] args) { Box myBox1=new Box(); Box myBox2=new Box(10,15,20); myBox1.volume(); myBox2.volume(); } }
Output of the Java program to create new class Box
Volume =0.0
Volume =3000.0
Explanation of Java Source Code
This Java program demonstrates the creation of a `Box` class and a `BoxDemo` class to show its usage. Let’s go through the code step by step:
1. Import Statements:
package boxdemo;
“`
The program uses the package named `boxdemo`. A package is a way to organize related classes together.
2. Box Class:
class Box {
private double width;
private double height;
private double depth;
“`
The `Box` class is defined with three private instance variables: `width`, `height`, and `depth`. These variables represent the dimensions of a 3D box and are accessible only within the `Box` class due to the `private` access modifier.
3. Constructors:
public Box() {
width = height = depth = 0.0;
}
public Box(double w, double h, double d) {
width = w;
height = h;
depth = d;
}
“`
The `Box` class has two constructors: a default constructor with no parameters and a parameterized constructor. The default constructor initializes all dimensions to zero, while the parameterized constructor allows you to set the dimensions when creating a `Box` object.
4. `volume` Method:
public void volume() {
System.out.println(“Volume =” + (width * height * depth));
}
“`
The `volume` method calculates and prints the volume of the box using the formula `width * height * depth`.
5. BoxDemo Class:
public class BoxDemo {
public static void main(String[] args) {
“`
The `BoxDemo` class contains the `main` method, which serves as the entry point of the program.
6. Creating Box Objects and Calling Methods:
Box myBox1 = new Box();
Box myBox2 = new Box(10, 15, 20);
“`
In the `main` method, two `Box` objects are created. `myBox1` uses the default constructor, and `myBox2` uses the parameterized constructor with the provided dimensions.
7. Calling `volume` Method:
myBox1.volume();
myBox2.volume();
“`
The `volume` method is called on both `myBox1` and `myBox2` objects, printing their respective volumes.
When you run this Java program, it will output the volumes of both boxes. The volume of `myBox1` will be 0.0 since it was initialized with zero dimensions, while the volume of `myBox2` will be calculated based on the dimensions provided in the constructor (10 * 15 * 20 = 3000).