Topic: Find Factorial by Recursive Function Python GUI Program
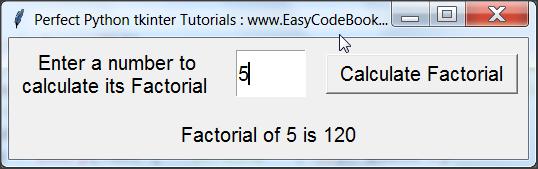
Find Factorial by Recursive Function Python GUI Program
# Python GUI program Find Factorial # of a Number by Recursion # Input a number and show its factorial # using a user defined recursive factorial function # www.EasyCodeBook.com from tkinter import * def inputn(): n= int(entry.get()) # call recursive_factorial function fact= recursive_factorial(n) str1 = "Factorial of " + str(n) + " is " + str(fact) output_label.configure(text = str1, justify=LEFT) def recursive_factorial(n): # We know that Base case is factorial of 0 is 1 # or 0! = 1 if n == 0: return 1 # We also Know that Recursive case is : n! = n * (n-1)! else: return n * recursive_factorial(n-1) main_window = Tk() main_window.title("Perfect Python tkinter Tutorials : www.EasyCodeBook.com") message_label = Label(text= ' Enter a number to \ncalculate its Factorial ' , font=( ' Verdana ' , 12)) output_label = Label(font=( ' Verdana ' , 12)) entry = Entry(font=( ' Verdana ' , 12), width=6) calc_button = Button(text= ' Calculate Factorial ' , font=( ' Verdana ', 12), command=inputn) message_label.grid(row=0, column=0,padx=10, pady=10) entry.grid(row=0, column=1,padx=10, pady=10, ipady=10) calc_button.grid(row=0, column=2,padx=10, pady=10) output_label.grid(row=1, column=0, columnspan=3,padx=10, pady=10) mainloop()
How this program works?
As we know a Recursive function is a function that normally calls itself. the process of calling a function itself is called Recursion.
Recursion may provide a concise solution to a problem that uses loops.
The factorial of a number say 3 is 1 x 2 x 3 that is 6.
Similarly the factorial of a number n is: 1 x 2 x 3 x…x(n-1) x n
We can say that n! = n * (n-1)!
Perfect Programming Tutorials, Python , Java, C Language, C++
You would also like Python GUI Programs using tkinter Module:
- Python GUI Temperature Conversion Program Celsius to Fahrenhiet
- Python GUI Program Temperature Conversion Fahrenheit to Celsius
- Python Pounds to Kilogram Converter GUI tkinter Program
- Python 3 Four Function Calculator Program tkinter GUI
- Python Digital Clock Program using tkinter GUI
- Python Quotes Changer Program tkinter GUI
- Python 3 tkinter Spinbox GUI Program Example
- Python 3 tkinter Message Widget Program Examples
- Python 3 GUI Program tkinter Radio Buttons
- Adding Menus to Python 3 tkinter GUI Programs
- Python 3 GUI Program Add Two Numbers
- Python 3 GUI Miles to Kilometers Converter tkinter Program
- Python 3 tkinter GUI Kilogram to Pounds Program
- Python Set Label Text on Button Click tkinter GUI Program
- Show Label and Button Widgets Python Tkinter Program
- Python GUI Program Area of Triangle with base and height
- Python 3 Tkinter GUI Hello World Program
- Create and Show Root Window of tkinter GUI Program
- Find Factorial of Number by Recursion Python GUI Program
- Multiplication Table Python GUI Program
Pingback: Python Spinbox Change Fontsize GUI Program | EasyCodeBook.com
Pingback: Python 3 Radio Buttons GUI Program | EasyCodeBook.com