Q: Write a C Program to Find Largest Number in Array.
The Basic Logic Behind Finding Maximum Number in Array
- First of all the user will input any 5 numbers in array.
- The program assumes that the first number is maximum number
- max = numArray[0];
- This C program uses a for loop to get each number in the given array and compare it with current maximum number. If the current item of array is greater than current maximum number, then assign current array item to max
- if( numArray[i] > max)
- max = numArray[i];
- After termination of loop the maximum number will be in max variable.
- Display the value of max variable
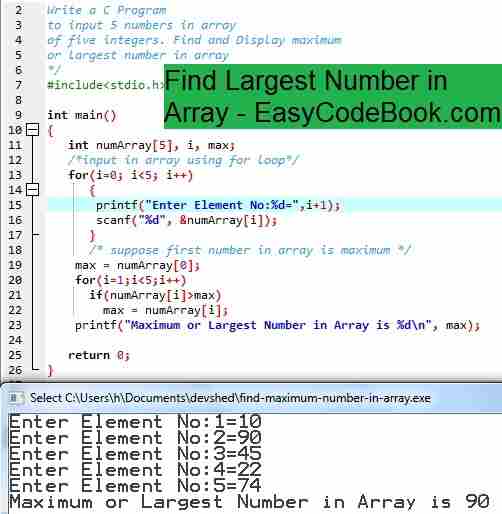
Write a C Program to Find Largest Number in Array.
The source code of C Program to find and show maximum number in array is:
/* Write a C Program to input 5 numbers in array of five integers. Find and Display maximum or largest number in array */ #include<stdio.h> int main() { int numArray[5], i, max; /*input in array using for loop*/ for(i=0; i<5; i++) { printf("Enter Element No:%d=",i+1); scanf("%d", &numArray[i]); } /* suppose first number in array is maximum */ max = numArray[0]; for(i=1;i<5;i++) if(numArray[i]>max) max = numArray[i]; printf("Maximum or Largest Number in Array is %d\n", max); return 0; }