Q: Write a C Program to Calculate Square Root of a Positive Number
How To Calculate Square Root of a number in C Programming
We use built-in function sqrt() to calculate square root of a number.
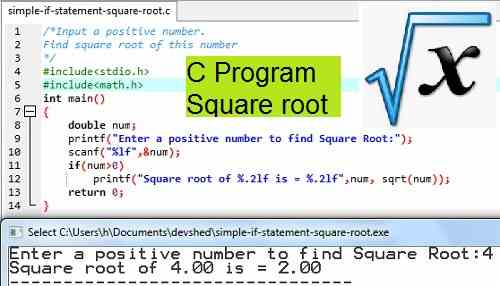
Include Header File math.h for sqrt() function
We must include math.h header file with the help of pre processor directive in the program. sqrt() function is defined in math.h header file.
Source Code for C Program to Find Square root of a number
/*Input a positive number. Find square root of this number */ #include<stdio.h> #include<math.h> int main() { double num; printf("Enter a positive number to find Square Root:"); scanf("%lf",&num); if(num>0) printf("Square root of %.2lf is = %.2lf",num, sqrt(num)); return 0; }
Explanation of Logic of this Program
In summary, the program prompts the user to enter a positive number, calculates its square root using the
sqrt
function from the math.h
library, and displays the result if the input is valid.- The program includes the necessary header files:
stdio.h
for input/output operations andmath.h
for mathematical functions. - The
main
function is defined as the entry point of the program. - Inside the
main
function, a variablenum
of typedouble
is declared to store the input number. - The
printf
function is used to display a prompt message asking the user to enter a positive number to find its square root. - The
scanf
function is used to read a double-precision floating-point number from the user and store it in thenum
variable.
- An
if
condition is used to check if the entered numbernum
is greater than 0, ensuring it is positive. - If the condition is true, the
printf
function is used to display the square root of the number. The%lf
format specifier is used to print the value ofnum
andsqrt(num)
with two decimal places. - The
sqrt
function from themath.h
library is used to calculate the square root ofnum
. - If the entered number is not positive, the program will skip the
printf
statement, and no output will be displayed.
- The
return 0;
is the last statement that indicates the successful termination of themain
function and the program.