Topic: Python 3 Four Function Calculator Program tkinter GUI
Widgets used in Simple 4 Function Calculator
We have used here:
- Label widgets to display labels for entry widgets for example. We use a label widget for showing result of addition, subtraction, multiplication or division. Last label simply displays ‘www.EasycodeBook.com for Python GUI Tutorials’.
- Two Entry widgets will receive user input. The user will enter two numbers in them.
- Four button widgets are used to perform 4 arithmetic functions of this calculator program
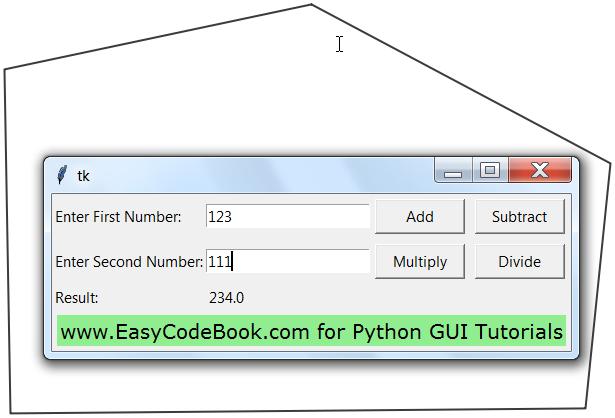
Python 3 Four Function Calculator Program tkinter GUI
# Simple 4 Function Calculator Program # using tkinter GUI module from tkinter import * def add_numbers(): res=float(e1.get())+ float(e2.get()) res = round(res,4) label_text.set(res) def subtract_numbers(): res=float(e1.get())- float(e2.get()) res = round(res,4) label_text.set(res) def multiply_numbers(): res=float(e1.get())* float(e2.get()) res = round(res,4) label_text.set(res) def divide_numbers(): res=float(e1.get()) / float(e2.get()) res = round(res,4) label_text.set(res) window = Tk() #window.geometry("400x300") label_text=StringVar(); Label(window, text="Enter First Number:").grid(row=0, column=0,sticky=W) Label(window, text="Enter Second Number:").grid(row=1,column=0, sticky=W) Label(window, text="Result:").grid(row=3,column=0, sticky=W) result=Label(window, text="", textvariable=label_text).grid(row=3,column=1, sticky=W) e1 = Entry(window) e2 = Entry(window) e1.grid(row=0, column=1) e2.grid(row=1, column=1) b1 = Button(window, text="Add", width=10,command=add_numbers) b1.grid(row=0, column=2, padx=5, pady=5,sticky=W) b2 = Button(window, text="Subtract", width=10,command=subtract_numbers) b2.grid(row=0, column=3, padx=5, pady=5,sticky=W) b3 = Button(window, text="Multiply", width=10,command=multiply_numbers) b3.grid(row=1, column=2, padx=5, pady=5,sticky=W) b4 = Button(window, text="Divide", width=10, command=divide_numbers) b4.grid(row=1, column=3, padx=5, pady=5,sticky=W) label_easycodebook = Label(text="www.EasyCodeBook.com for Python GUI Tutorials", bg='lightgreen',font=('verdana',12)) label_easycodebook.grid(row=5,column=0,padx=5,pady=5,columnspan=4) window.mainloop()
How Python 4 Function Calculator Program Works
Here we have defined the four user defined functions to perform addition,
subtraction, multiplication and division of the two numbers in the
text boxes.
def add_numbers():
res=float(e1.get())+ float(e2.get())
res = round(res,4)
label_text.set(res)
def subtract_numbers():
res=float(e1.get())- float(e2.get())
res = round(res,4)
label_text.set(res)
def multiply_numbers():
res=float(e1.get())* float(e2.get())
res = round(res,4)
label_text.set(res)
def divide_numbers():
res=float(e1.get()) / float(e2.get())
res = round(res,4)
label_text.set(res)
[
create 4 label widgets and using grid()
place them in window
][
create 2 entry widgets for input and using grid()
place them in window
create 4 button widgets and using grid()
place them in window
Use command=event_handler_function for click event
example:
b1 = Button(window, text=”Add”, width=10,command=add_numbers)
]
- Use Switch statement for simple calculator C Program
- Four Function Calculator Program in C programming
- Employee Salary with Bonus Calculation C++ Program
- C Program Sum and Average Calculator
- Java Program to Calculate Area of Circle
- C Program To Calculate Circumference of Circle
Pingback: Multiplication Table Python GUI Program | EasyCodeBook.com
Pingback: Find Factorial by Recursive Function Python GUI Program | EasyCodeBook.com
Pingback: Python Quotes Changer Program tkinter GUI | EasyCodeBook.com
Pingback: Python Spinbox Change Fontsize GUI Program | EasyCodeBook.com