Q: Write a C Program To Calculate Circumference of Circle. Input radius of the circle from the user at run time. Use the formula:
circumference of circle = 2 * PI * radius
The Logic Behind C Program to Find Circumference of Circle
This is a simple C program using formula calculation technique. The basic formula calculation involves writing C expressions.
Convert Circumference of Circle Formula into C Expression
The formula to find circumference of the circle is given as:
circumference of circle = 2 x PI x R
where PI is a popular mathematical constant having the value 3.1415.
And R stands for radius of the circle.
We convert this formula to C expression as under:
circumference = 2 * PI * radius;
How to define constant PI in a C Program
We may use #define pre processor directive to dfine a constant in C language, normally before the start of main() function.
Syntax of #define To deifine a Constant in C Program
#define NMAE value
Example:
#define PI 3.1415
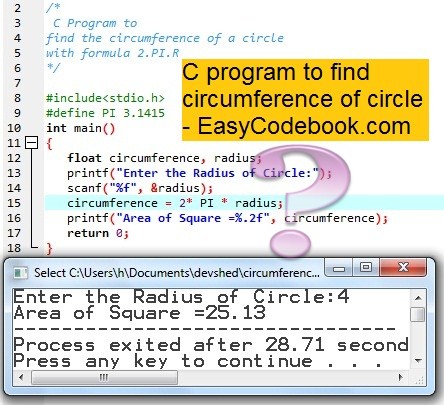
The source code of C program to compute circumference of circle
/* C Program to find the circumference of a circle with formula 2.PI.R */ #include<stdio.h> #define PI 3.1415 int main() { float circumference, radius; printf("Enter the Radius of Circle:"); scanf("%f", &radius); circumference = 2* PI * radius; printf("Area of Square =%.2f", circumference); return 0; }