Python PyQt Weight Converter Pounds and Kilograms – This code creates a PyQt application with two radio buttons for selecting the conversion type, updates the labels accordingly, and performs the conversions based on the selected option. It assumes the conversion factors are 0.45359237 for pounds to kilograms and 2.20462 for kilograms to pounds.
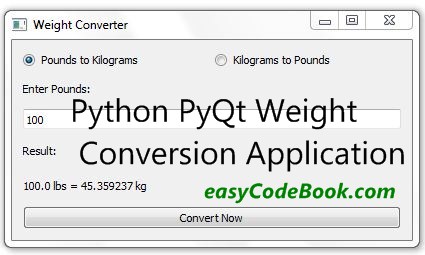
Python PyQt Weight Converter Pounds and Kilograms
This code includes a “Convert Now” button, and when you click it, it will perform the conversion based on the selected radio button option and display the result in the result label.
import sys from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel, QLineEdit, QPushButton, QRadioButton, QHBoxLayout class ConverterApp(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle('Weight Converter') self.setGeometry(100, 100, 400, 200) self.input_label = QLabel('Enter Pounds:') self.input_box = QLineEdit(self) self.result_label = QLabel('Result:') self.result_box = QLabel(self) self.radio_pounds_to_kg = QRadioButton('Pounds to Kilograms', self) self.radio_kg_to_pounds = QRadioButton('Kilograms to Pounds', self) self.radio_pounds_to_kg.setChecked(True) self.convert_button = QPushButton('Convert Now', self) layout = QVBoxLayout() radio_layout = QHBoxLayout() radio_layout.addWidget(self.radio_pounds_to_kg) radio_layout.addWidget(self.radio_kg_to_pounds) layout.addLayout(radio_layout) layout.addWidget(self.input_label) layout.addWidget(self.input_box) layout.addWidget(self.result_label) layout.addWidget(self.result_box) layout.addWidget(self.convert_button) self.setLayout(layout) self.radio_pounds_to_kg.toggled.connect(self.update_labels) self.radio_kg_to_pounds.toggled.connect(self.update_labels) self.convert_button.clicked.connect(self.convert) def update_labels(self): if self.radio_pounds_to_kg.isChecked(): self.input_label.setText('Enter Pounds:') self.result_label.setText('Result (Kilograms):') else: self.input_label.setText('Enter Kilograms:') self.result_label.setText('Result (Pounds):') self.input_box.clear() self.result_box.clear() def convert(self): try: value = float(self.input_box.text()) if self.radio_pounds_to_kg.isChecked(): result = value * 0.45359237 # Pounds to Kilograms conversion self.result_box.setText(f'{value} lbs = {result} kg') else: result = value * 2.20462 # Kilograms to Pounds conversion self.result_box.setText(f'{value} kg = {result} lbs') except ValueError: self.result_box.setText('Invalid input') if __name__ == '__main__': app = QApplication(sys.argv) converter = ConverterApp() converter.show() sys.exit(app.exec_())
Sample Output
To enter 100 pounds and convert it to kilograms using the PyQt application provided, follow these steps:
- Run the PyQt application: Make sure you have the application code running in your Python environment. If you haven’t already, run the code.
- Launch the application: When the application is running, a GUI window should appear with two radio buttons: “Pounds to Kilograms” and “Kilograms to Pounds.” The “Pounds to Kilograms” option should be selected by default.
- Enter the value: In the “Enter Pounds” input box, click to select it, and type “100” to represent 100 pounds.
- Convert the value: Click the “Convert Now” button. The application will process your input and convert 100 pounds to kilograms based on the selected radio button option. In this case, it will convert it to kilograms.
- View the result: The result will be displayed in the “Result (Kilograms)” label. You should see the converted value, which is 45.359237 kilograms.
The conversion factor used in this application is 0.45359237, which is the factor to convert pounds to kilograms. So, 100 pounds * 0.45359237 = 45.359237 kilograms.