Python Mini Ludo 20 Game – This PyQt-based game allows two players to take turns, roll dice, and compete to reach position 20. It provides a simple and interactive way to play a virtual Ludo-style game.
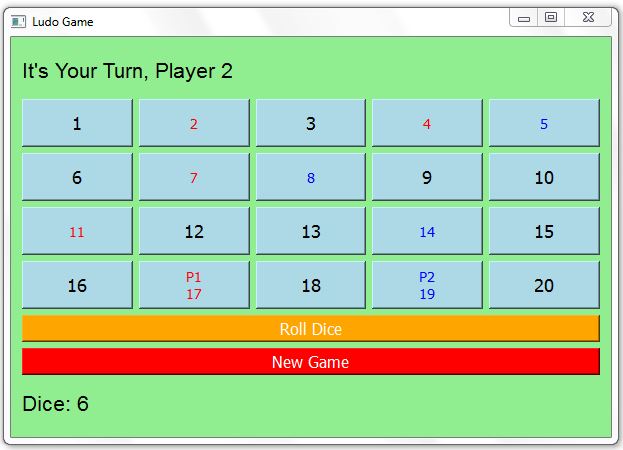
Python Mini Ludo 20 Game in PyQt
Source Code
import sys import random from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout, QHBoxLayout, QLabel, QMessageBox from PyQt5.QtGui import QFont class LudoGame(QWidget): def __init__(self): super().__init__() self.init_ui() def init_ui(self): self.setWindowTitle("Ludo Game") self.setGeometry(100, 100, 600, 400) self.setStyleSheet("background-color: lightgreen;") self.buttons = [None] * 20 self.current_player = 1 self.position_p1 = 0 self.position_p2 = 0 main_layout = QVBoxLayout() self.turn_label = QLabel("It's Your Turn, Player 1", self) self.turn_label.setFont(QFont("Arial", 16)) main_layout.addWidget(self.turn_label) board_layout = QVBoxLayout() row_layout = QHBoxLayout() for num in range(1, 21): button = QPushButton(f"{num}", self) button.setStyleSheet("font-size: 18px; height: 40px; background-color: lightblue; color: black") button.clicked.connect(lambda state, button=button, num=num: self.move_player(button, num)) self.buttons[num - 1] = button row_layout.addWidget(button) if (num % 5) == 0: board_layout.addLayout(row_layout) row_layout = QHBoxLayout() main_layout.addLayout(board_layout) roll_button = QPushButton("Roll Dice", self) roll_button.clicked.connect(self.roll_dice) roll_button.setStyleSheet("font-size: 16px; background-color: orange; color: white") main_layout.addWidget(roll_button) new_game_button = QPushButton("New Game", self) new_game_button.clicked.connect(self.new_game) new_game_button.setStyleSheet("font-size: 16px; background-color: red; color: white") main_layout.addWidget(new_game_button) self.dice_label = QLabel("", self) self.dice_label.setFont(QFont("Arial", 16)) main_layout.addWidget(self.dice_label) self.setLayout(main_layout) def roll_dice(self): dice_result = random.randint(1, 6) self.dice_label.setText(f"Dice: {dice_result}") self.move_player(self.buttons[self.current_player_position()], dice_result) def move_player(self, button, steps): if self.check_winner(): return current_position = self.current_player_position() self.buttons[current_position].setText(str(current_position + 1)) if self.current_player == 1: self.position_p1 += steps self.position_p1 = min(self.position_p1, 19) self.move_player_on_board(self.position_p1, "P1") else: self.position_p2 += steps self.position_p2 = min(self.position_p2, 19) self.move_player_on_board(self.position_p2, "P2") if self.check_winner(): return self.toggle_player() def toggle_player(self): self.current_player = 1 if self.current_player == 2 else 2 self.turn_label.setText(f"It's Your Turn, Player {self.current_player}") def move_player_on_board(self, position, player): self.buttons[position].setText(f"{player}\n{position + 1}") self.buttons[position].setStyleSheet("font-size: 14px; height: 40px; background-color: lightblue; color: red" if player == 'P1' else "font-size: 14px; height: 40px; background-color: lightblue; color: blue") def current_player_position(self): return self.position_p1 if self.current_player == 1 else self.position_p2 def check_winner(self): if self.position_p1 >= 19: self.announce_winner("Player 1 (P1)") return True elif self.position_p2 >= 19: self.announce_winner("Player 2 (P2)") return True return False def announce_winner(self, player): QMessageBox.information(self, 'Ludo Game', f'{player} wins!') def new_game(self): self.position_p1 = 0 self.position_p2 = 0 self.current_player = 1 self.turn_label.setText("It's Your Turn, Player 1") self.dice_label.setText("") for i in range(20): self.buttons[i].setText(f"{i + 1}") self.buttons[i].setStyleSheet("font-size: 18px; height: 40px; background-color: lightblue; color: black") if __name__ == '__main__': app = QApplication(sys.argv) window = LudoGame() window.show() sys.exit(app.exec_())
ThisĀ version of the Ludo-style game in PyQt includes several features:
- Player Labels: At the top of the game window, there is a label that displays “It’s Your Turn, Player 1” initially. This label keeps track of the current player’s turn.
- Board Buttons: The game board consists of 20 buttons labeled from 1 to 20. These buttons represent the positions where the players’ pieces will move.
- Roll Dice Button: There is a “Roll Dice” button that simulates rolling a six-sided die. When you click this button, a random number between 1 and 6 is generated and displayed as “Dice: [number]” below the board.
- Player Movements: When you roll the dice, the current player’s piece (either ‘P1’ or ‘P2’) moves forward on the board by the number rolled. The piece is displayed on the button with the player’s symbol and the button number.
- Switching Players: After a player’s turn, the game automatically switches to the other player. The player labels are updated accordingly.
- Winning Condition: If a player’s piece reaches or goes beyond position 20, a message box appears announcing that player as the winner. The game then ends, and you can start a new game.
- New Game Button: You can click the “New Game” button to reset the game and start over with both players at position 1. The turn resets to Player 1.
- Styling: The game uses various styles to make it visually appealing, such as changing the background color, button colors, and font sizes.
This PyQt-based game allows two players to take turns, roll dice, and compete to reach position 20. It provides a simple and interactive way to play a virtual Ludo-style game.